How to reverse the letters in a string in Java
In this article, we'll learn various ways to reverse strings in Java. An algorithmic approach with a flow chart and steps. Using java API methods to solve this problem and rotate kth elements or characters in an array.
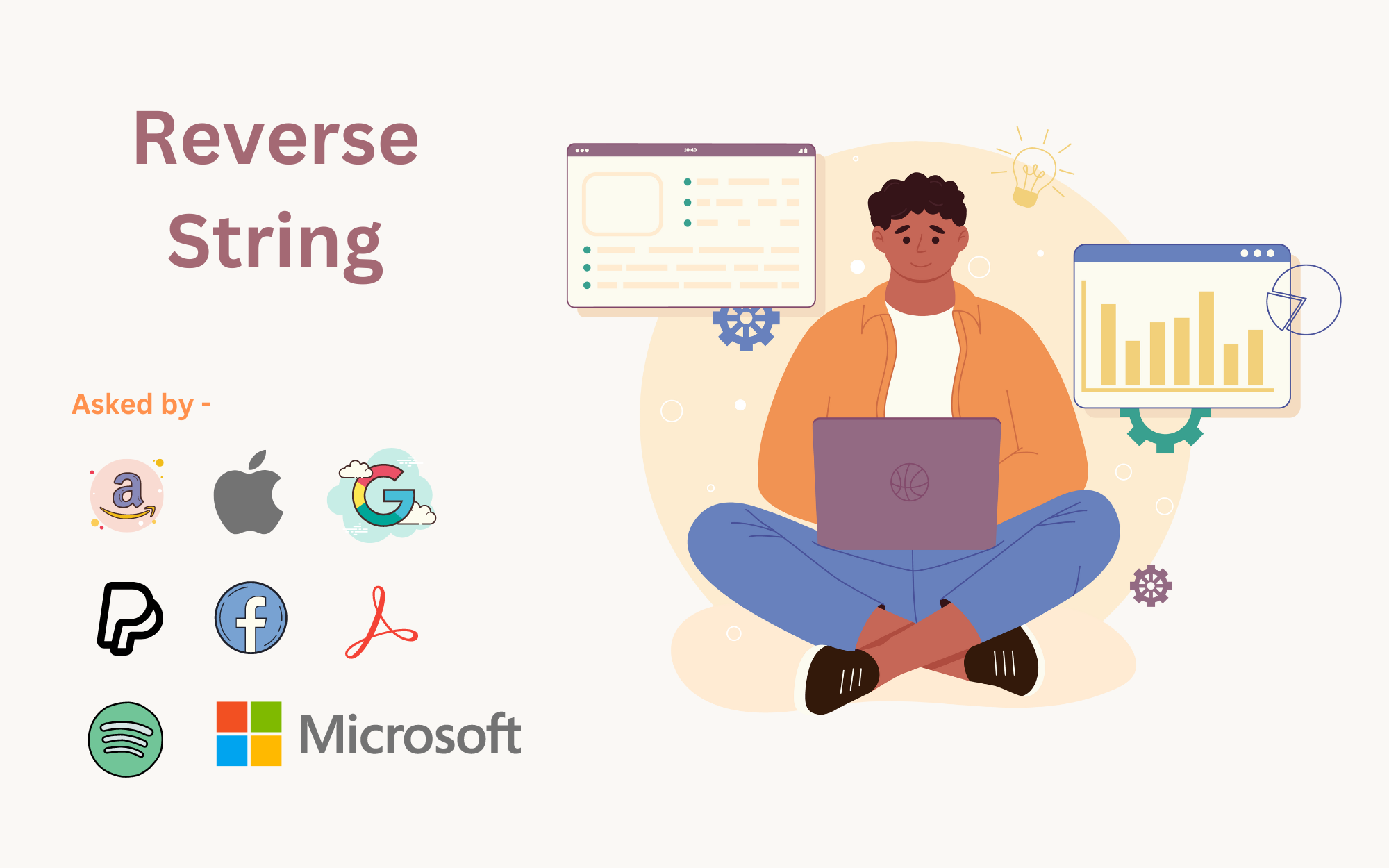