Spring Boot Hello World Tutorial with Lombok and H2 Database – Quick Start for Beginners
Learn how to create a Hello World Spring Boot application using Lombok for cleaner code and the H2 in-memory database for rapid development. This step-by-step guide includes annotations, project setup, REST API, H2 console access, and more to kickstart your Spring Boot journey.
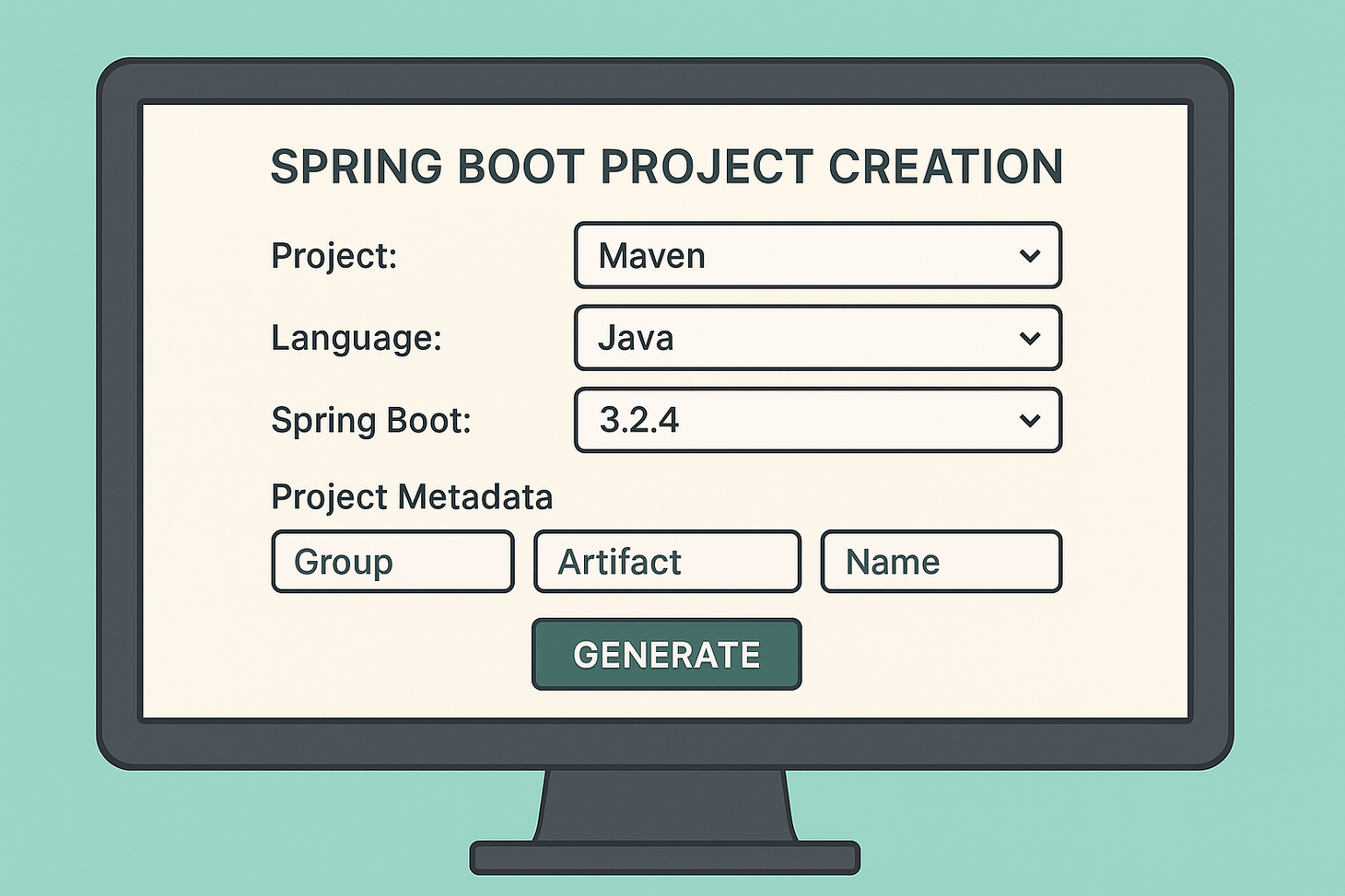
How to fix port 8080 already in use error on Windows and macOS
Getting the “Web server failed to start. Port 8080 was already in use” error? Learn how to identify and kill the process blocking port 8080 on Windows and macOS using simple terminal commands. Fix server startup issues fast and keep your development running smoothly.
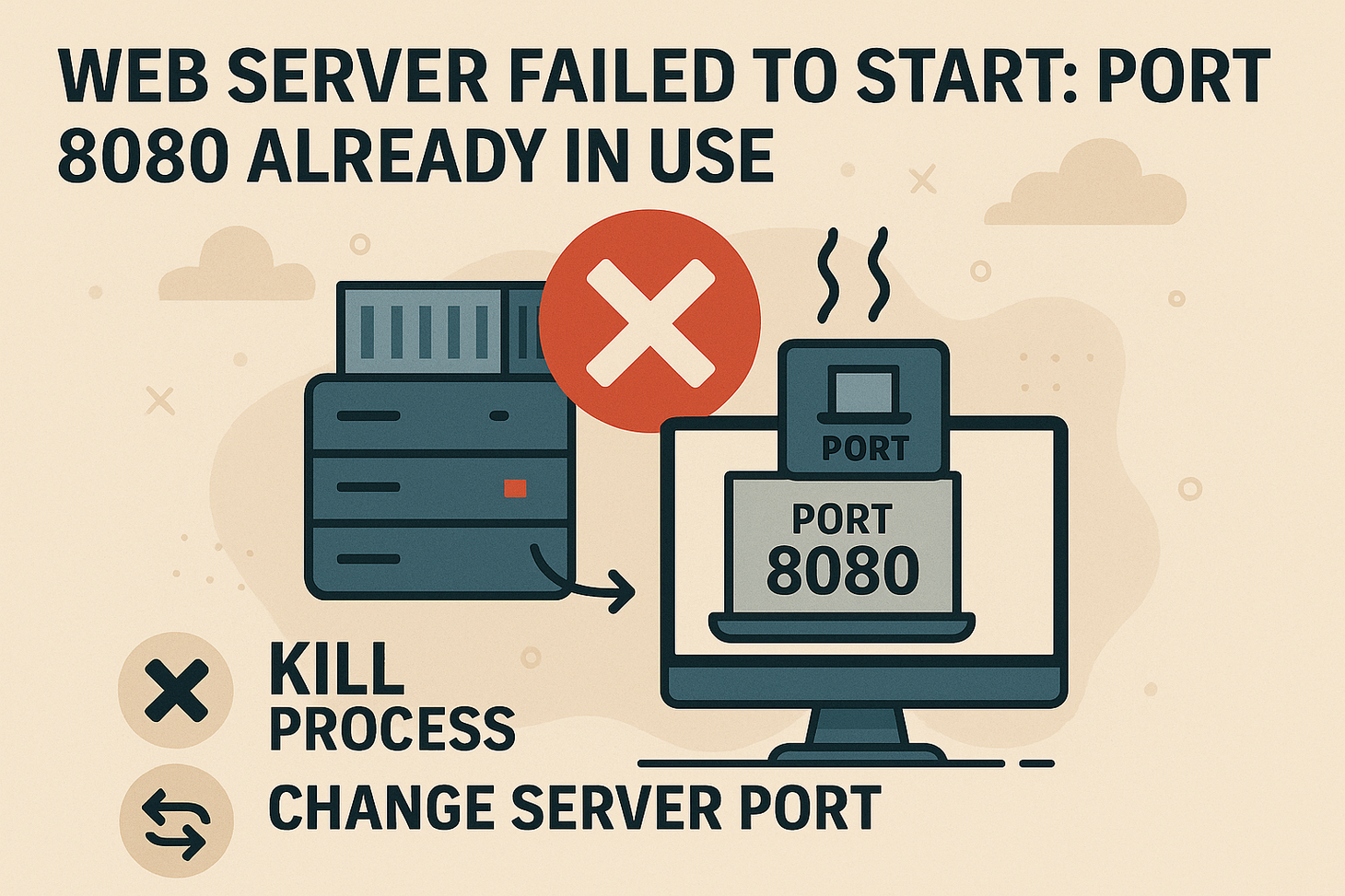
Spring vs. Spring Boot: Key Differences Developers Must Know
Spring or Spring Boot? One gives you full control, the other gets you up and running fast. If you're building Java apps, knowing the difference isn't optional—it’s critical. Here’s the breakdown every dev should read.
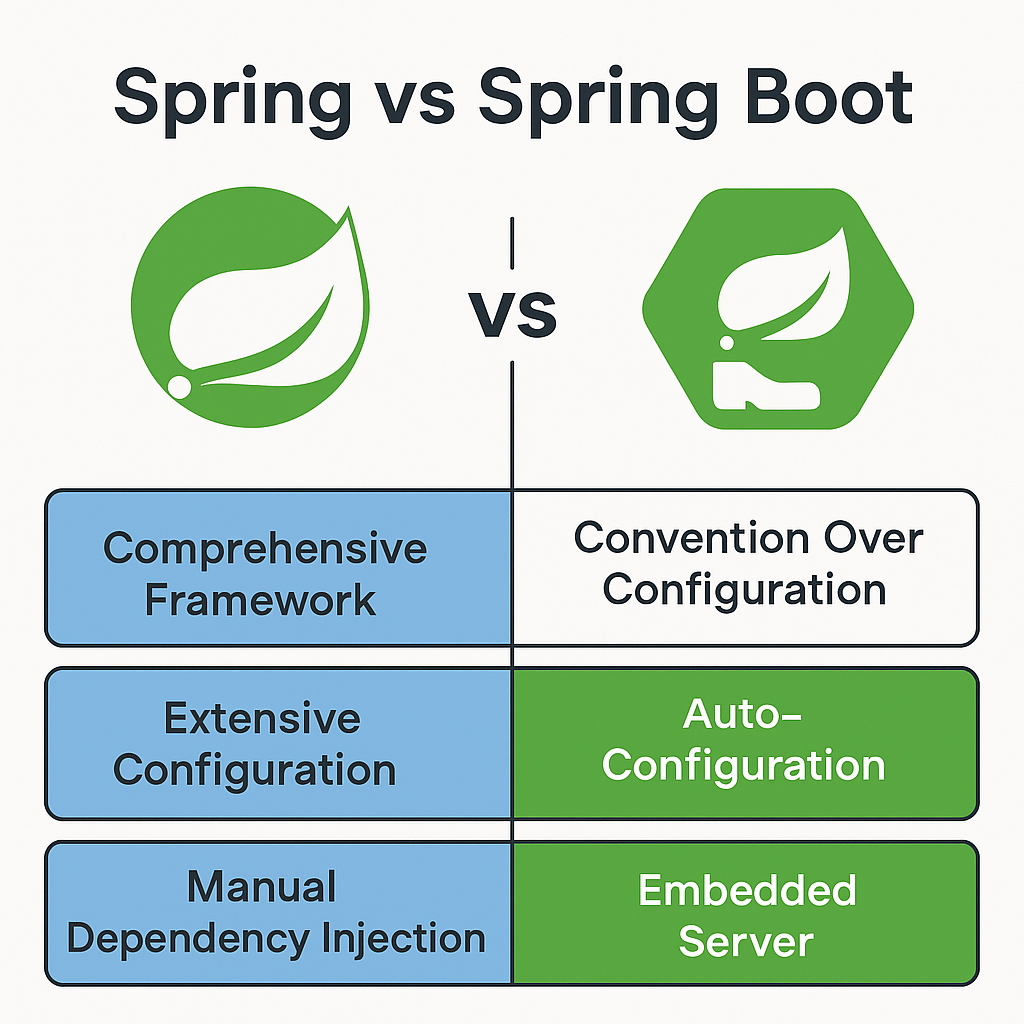
NoSuchBeanDefinitionException: The Most Common Spring Bean Error
Learn how to fix NoSuchBeanDefinitionException in Spring Boot with clear examples and best practices for dependency injection, package scanning, and bean registration.
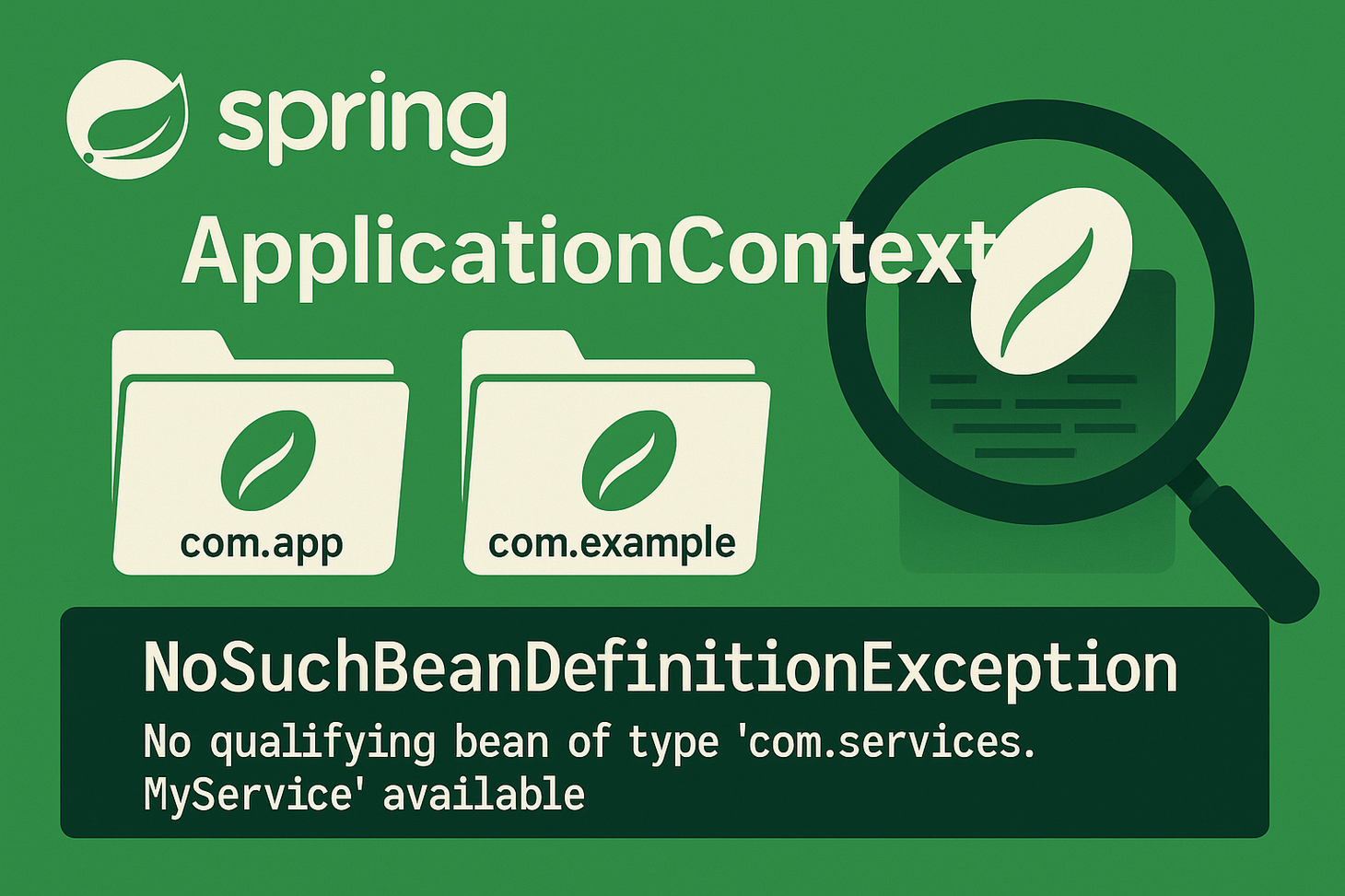
What are the different approaches or styles in designing and building APIs?
REST, SOAP, GraphQL, and gRPC are some approaches or styles in designing and building APIs. This lesson talks about each of them in detail with tabulation comparison.
What is an API?
APIs are tools that allow two software programs to talk to each other by following specific rules and guidelines.
Byte Primitive Type in Java
Understanding byte is essential, especially when working with large amounts of data or optimizing your program's memory usage. We'll cover its size, range, use cases, and provide example usage to solidify your understanding. What is the byte Type? In Java, byte is one of the eight
Memory Allocation: Stack vs. Heap
Understanding where and how primitive types are stored in memory is crucial for writing efficient Java programs. Java uses two main types of memory allocation: stack and heap. Memory allocations Stack Memory What is the Stack? The stack is a region of memory that stores local variables and method calls.
What Are Primitive Data Types In Java?
Introduction Data types in Java are divided into two types that are used to define variables include: 1. Primitive data types 2. Non-primitive (or reference) data types. Sketch The following sketch helps you understand how everything is divided based on its type. Primitive data types In Java, most of the