Subsets Or Powerset
In subsets or powerset problem, we need to write a program that finds all possible subsets (the power set) of a given input. The solution set must not contain duplicate elements.
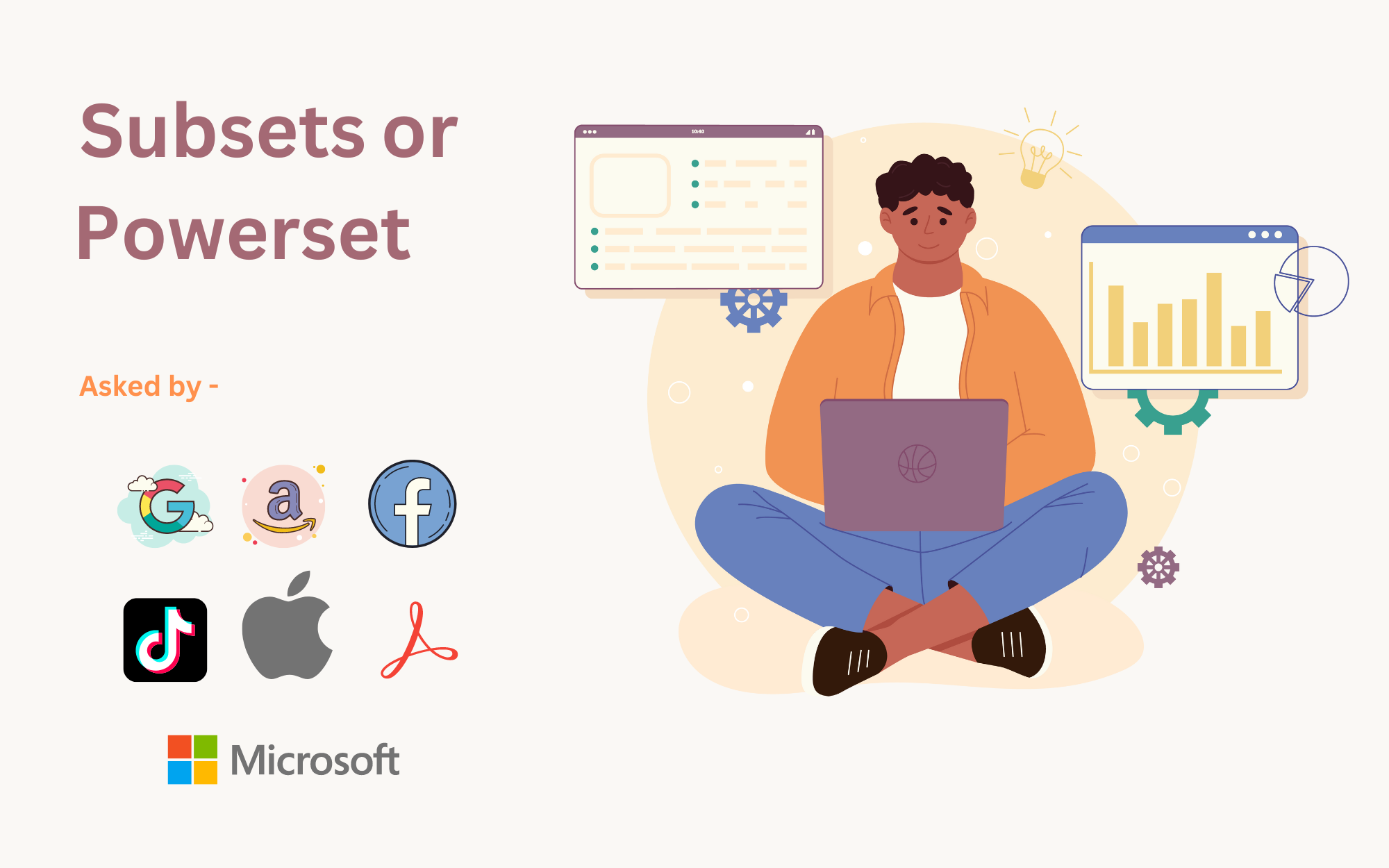
Course Overview "Arrays Mastery For Coding Interviews" is a comprehensive program designed to provide an in-depth understanding of arrays, a fundamental data structure in computer science. The course covers various topics, including array manipulation, multi-dimensional arrays, dynamic arrays, and common algorithms. Students will learn through theoretical lessons and
This lesson will teach you how to find the duplicate element using a hashing algorithm.