Leetcode 1: Two Sum Problem
This lesson on "Two Sum" coding problem is discussed thoroughly with illustrations and various approaches with running time complexities.
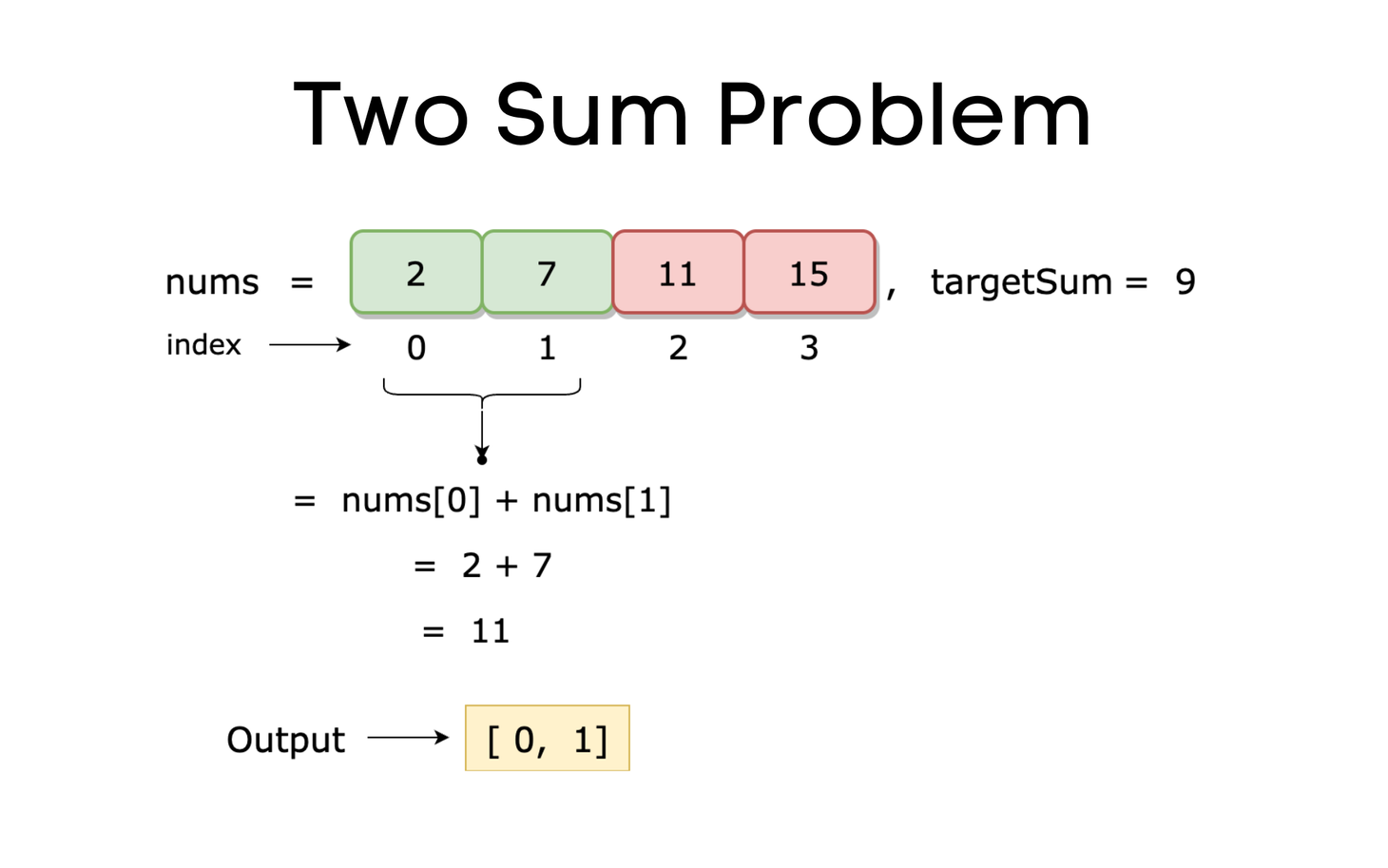
Course Overview "Arrays Mastery For Coding Interviews" is a comprehensive program designed to provide an in-depth understanding of arrays, a fundamental data structure in computer science. The course covers various topics, including array manipulation, multi-dimensional arrays, dynamic arrays, and common algorithms. Students will learn through theoretical lessons and
This lesson will teach you how to find the duplicate element using a hashing algorithm.