What Are Wrapper Classes in Java?
Table of Contents
This is the most asked interview question! Why do we use wrapper classes to store data for a model class instead of primitives?
What are wrapper classes?
Primitive wrappers, also known as wrapper classes, are a set of classes in Java that provide an object representation for the primitive data types.
Wrapper classes are all final, so once created, you cannot alter the underlying value. They are immutable. Autoboxing and unboxing use value copy. There are no shared values or references. Every boxing operation is a copy.
They allow you to treat primitive types as objects. This is necessary in situations where you need to use objects rather than primitives, such as in collections (like ArrayList
or LinkedList
) or when you want to take advantage of object-oriented features like inheritance and polymorphism.
Wrapper classes are part of the java.lang
package and are automatically imported into any Java program, so you don't need to import them explicitly.
Sketch / Illustration
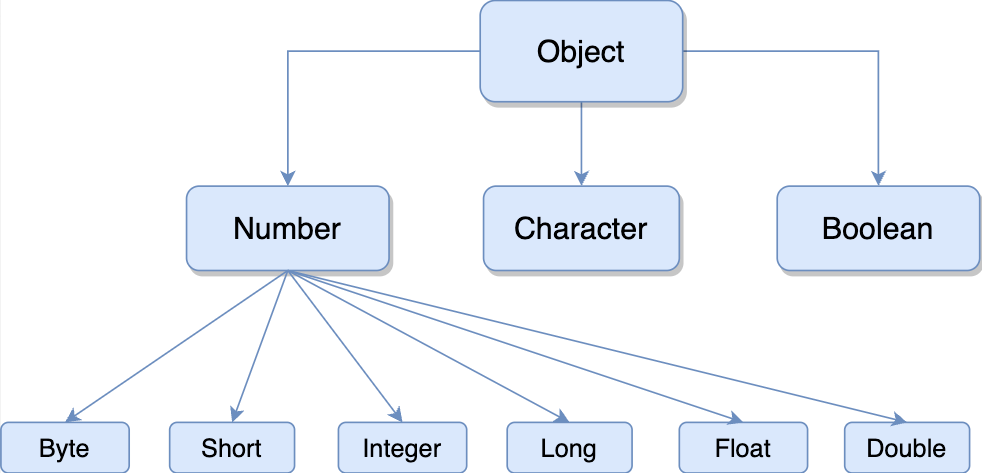
Boolean
and Character
are subtypes of the Object
class.
But the other primitive wrappers Byte
, Short
, Integer
, Long
, Float
, and Double
are all subtypes of Number
class.
Primitives & their wrappers
There is a wrapper class available for each of the primitive types.
- Integer types
byte
-> The wrapper isjava.lang.Byte
.short
-> The wrapper isjava.lang.Short
.int
-> The wrapper isjava.lang.Integer
.long
-> The wrapper isjava.lang.Long
.
- Floating point types
float
-> The wrapper isjava.lang.Float
.double
-> The wrapper isjava.lang.Double
.
- Character type
char
-> The wrapper isjava.lang.Character
.
- Boolean type
boolean
-> The wrapper isjava.lang.Boolean
.
🔥 Why Use Wrapper Classes?
Wrapper classes serve several purposes, including:
- Facilitating use in Java generics: Generic types in Java can only work with objects, not primitive types. Wrapper classes enable you to use primitive types in generic classes.
- Allowing primitive types to be used as objects: In situations where objects are expected (e.g., collections, generics, or method parameters), wrapper classes enable you to use primitive types.
- Providing utility methods: Wrapper classes provide methods for converting, parsing, and manipulating primitive values. For example,
Integer
class provides methods likeparseInt()
,toString()
, etc. - Supporting null values: Primitive data types cannot represent null values, but wrapper classes can, allowing you to represent the absence of a value.
Auto-Boxing and Auto-Unboxing
Java also provides a feature called auto-boxing and auto-unboxing. This allows the conversion between primitive types and their corresponding wrapper classes to happen automatically when needed.
Auto-boxing
The process of converting a primitive type to its corresponding wrapper class automatically. For example, int
to Integer
.
// Autoboxing: converting int to Integer
int primitiveInt = 10;
Integer wrappedInt = primitiveInt; // Autoboxing
Behind the scenes, this is equivalent to explicitly creating an Integer
object like this:
Integer wrappedInt = new Integer(primitiveInt);
Auto-unboxing
The process of converting a wrapper class object to its corresponding primitive type automatically. For example, Integer
to int
.
From Integer
to int
.
// Unboxing: converting Integer to int
Integer wrappedInt = 20;
int primitiveInt = wrappedInt; // Unboxing
These features make it more convenient to work with both primitive types and their wrapper classes in Java.
Example
public class WrapperExample {
public static void main(String[] args) {
// Creating instances of wrapper classes
Integer intWrapper = new Integer(10); // Deprecated in Java 9 and removed in Java 11
Integer intWrapperNew = Integer.valueOf(10); // Preferred way to create Integer objects
Double doubleWrapper = new Double(3.14);
Character charWrapper = new Character('A');
Boolean boolWrapper = new Boolean(true);
// Autoboxing (converting primitive to wrapper)
Integer anotherIntWrapper = 20; // Autoboxing - int to Integer
Double anotherDoubleWrapper = 5.0; // Autoboxing - double to Double
Boolean anotherBoolWrapper = true; // Autoboxing - boolean to Boolean
// Unboxing (converting wrapper to primitive)
int primitiveInt = intWrapperNew.intValue(); // Unboxing - Integer to int
double primitiveDouble = doubleWrapper.doubleValue(); // Unboxing - Double to double
char primitiveChar = charWrapper.charValue(); // Unboxing - Character to char
// Using wrapper classes in collections
ArrayList<Integer> intList = new ArrayList<>();
intList.add(30); // Autoboxing - int to Integer
int value = intList.get(0); // Unboxing - Integer to int
// Wrapper classes can be helpful when working with APIs that require objects, such as collections or generics.
}
}
Additional resources
Gopi Gorantala Newsletter
Join the newsletter to receive the latest updates in your inbox.