Java Variables and Data Types
This is an introductory lesson on variables or different data types available in Java.
Table of Contents
What are variables?
In order to do anything interesting in our programs, we need a way to store and manipulate values, that's where variables come in. A variable is simply a named area of storage.
Variables store data but what kind of data a variable is storing? This arises a problem and introduces a concept called "data type"( or the type of data).
It's important to note that Java is a strongly-typed language, which means that variables must be declared with a specific data type before they can be used. This helps to ensure that the code is more reliable and less prone to errors.
Data types
In Java, a data type is a classification of data that determines the type of values a variable can hold and the operations that can be performed on it.
Java data types are used to define variables that store different types of values, such as numbers, characters, and boolean values, and they play a crucial role in ensuring that programs are reliable, efficient, and free of errors.
Syntax
The syntax of declaring a variable with a specific data type is as follows:
<data_type> <variable_name>;
For example, to declare a variable named "age" with an integer data type, the syntax would be:
int age;
age
is an un-initialized variable, it means it's been declared but does not yet have a value.
Data types in Java are divided into two types that are used to define variables include:
- Primitive data types
- Non-primitive (or reference) data types.
It's important to note that Java is a strongly-typed language, which means that variables must be declared with a specific data type before they can be used. This helps to ensure that the code is more reliable and less prone to errors.
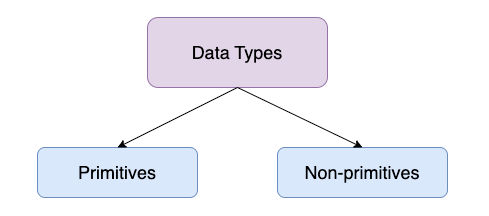
Primitive data type
Primitive data types are the most basic data types in Java, and they are used to store simple values like numbers and characters.
Primitive data types are stored directly in memory and are accessed faster than non-primitive data types.
They are also passed by value, meaning that when they are passed as arguments to a method or assigned to a variable, a copy of their value is made and passed/assigned, and any changes made to the copy do not affect the original value.
Syntax
To initialize the variable with a value, the syntax would be:
<data_type> <variable_name> = <value>;
For example, to initialize the "age" variable to 25, the syntax would be:
int age = 25;
There are 8 primitive data types in Java.
boolean
: represents a boolean value, either true or false.byte
: represents an 8-bit integer value.short
: represents a 16-bit integer value.int
: represents a 32-bit integer value.long
: represents a 64-bit integer value.float
: represents a 32-bit floating point value.double
: represents a 64-bit floating point value.char
: represents a 16-bit Unicode character.
Reference data type
Non-primitive (or reference) data types, on the other hand, are more complex data types that are built from primitive data types, other non-primitive data types, or a combination of both. They include:
- Class: represents a class definition and is used to create objects.
- Array: represents a collection of elements of the same data type.
- Interface: represents a set of methods that a class must implement.
- String: represents a sequence of characters.
Syntax
Note that for non-primitive data types, the syntax is slightly different, as they are declared using class or interface names. For example, to declare a variable named person
with a Person
class type, the syntax would be:
Person person;
And to initialize it with a new instance of the Person
class, the syntax would be:
Person person = new Person();
where Person()
is the constructor method for the Person
class.
Note: You will understand more about class, constructors in next chapter.
Gopi Gorantala Newsletter
Join the newsletter to receive the latest updates in your inbox.