Introduction to Java Enums
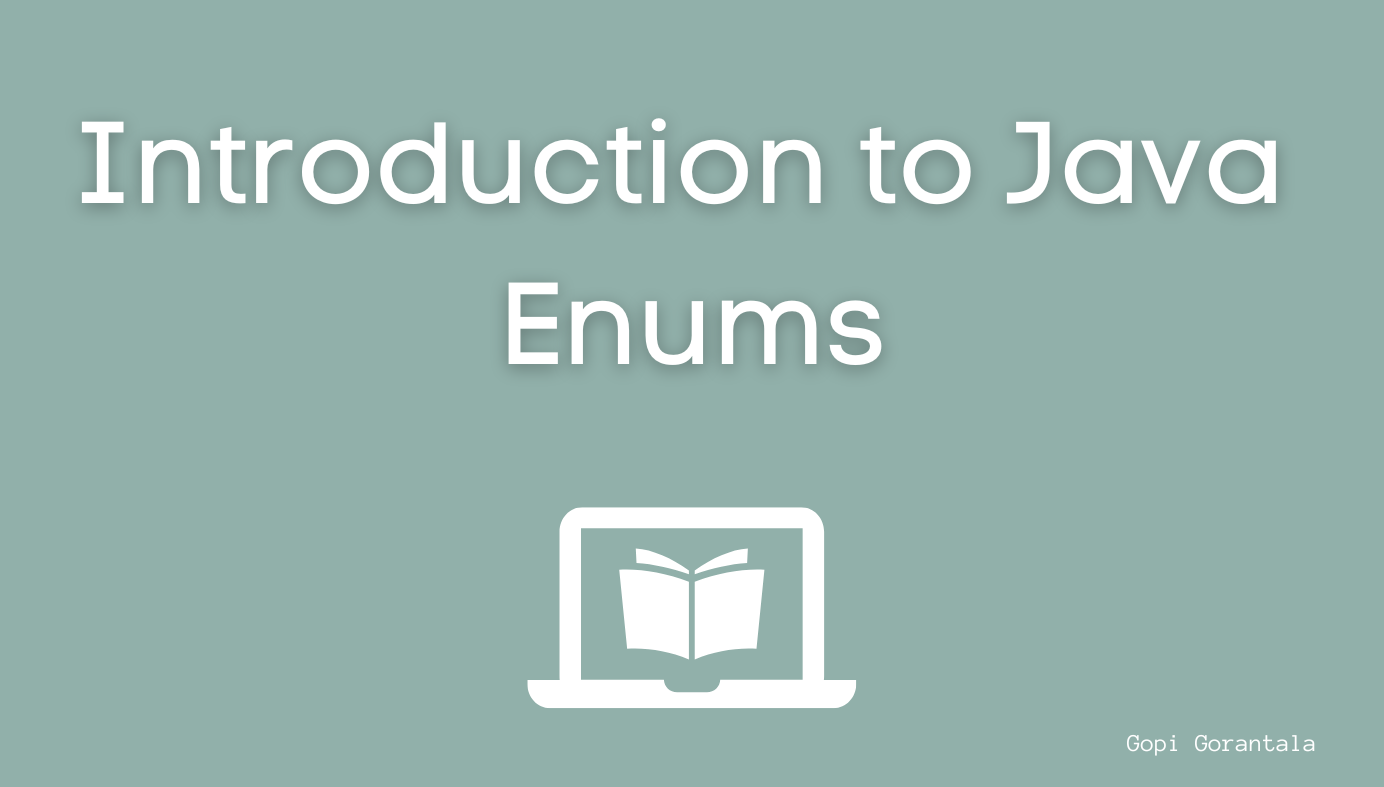
Table of Contents
Definition of enums
In Java, an enum (short for "enumeration") is a special data type that allows developers to define a set of named constants grouped under a single type.
Each constant represents a unique value that can be assigned to a variable of that enum type.
Enums were introduced in Java 5 to provide a more type-safe and robust alternative to traditional integer constants, which can be error-prone and difficult to maintain. Enums are also more expressive and self-documenting, making code more readable and easier to understand.
To define an enum in Java, you use the enum keyword followed by the name of the enum type and a list of the constant values enclosed in curly braces. For example, to define an enum for the days of the week, you could write:
enum Day {
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY,
SUNDAY
}
Each constant value in the enum is automatically assigned an ordinal value, starting from 0 for the first constant and incrementing by 1 for each subsequent constant. Using a constructor and fields, you can also assign custom values to each constant.
Enums can be used like any other data type in Java, passed as arguments to methods, and used in switch statements. Because enums are typesafe, the compiler can detect errors at compile time if an incorrect value is used.
Advantages of using enums
There are several advantages of using enums in Java:
1. Type safety: Enums provide a way to create a set of named constants with a specific type, which helps to avoid common programming errors, such as passing the wrong type of value or using an undefined constant.
2. Readability: By using enums, you can give meaningful names to the constants, making the code more readable and self-explanatory. It is often easier to understand code that uses enums rather than a bunch of integer or string constants.
3. Code maintainability: Enums can make your code easier to maintain by centralizing all the related constants in a single location. When a new constant needs to be added or an existing one is changed, you only need to update the enum definition rather than searching for all the constant occurrences throughout the codebase.
4. Enum methods: Enums can have associated methods, making the code more modular and easier to extend.
5. Compile-time checking: Since the values of an enum are fixed and known at compile time, the compiler can check that the values used in the code are valid. This can help detect errors at compile time rather than at runtime.
Using enums can make your code more robust, maintainable, and easily understood.
Enum syntax and declaration
In Java, the syntax for defining an enum is as follows:
enum EnumName {
CONSTANT1,
CONSTANT2,
...
}
EnumName is the enum's name, and CONSTANT1, CONSTANT2, and so on are the named constants that belong to the enum. The list of constants is separated by commas and enclosed in curly braces.
Each named constant is declared using the enum syntax, which looks like this:
CONSTANT_NAME
For example, here is an example of an enum for days of the week:
enum DayOfWeek {
SUNDAY,
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY
}
This defines an enum named DayOfWeek with seven named constants. Each constant is just a name, and by default, it has an integer value starting from zero that corresponds to its position in the enum.
Like classes, enums can also have constructors, fields, and methods. You can define them after the list of constants, separated by semicolons. For example:
enum Color {
RED(255, 0, 0),
GREEN(0, 255, 0),
BLUE(0, 0, 255);
private int r, g, b;
Color(int r, int g, int b) {
this.r = r;
this.g = g;
this.b = b;
}
public int getR() {
return r;
}
public int getG() {
return g;
}
public int getB() {
return b;
}
}
In this example, the enum Color has three named constants RED, GREEN, and BLUE, each with its RGB value. The constructor takes the three values as arguments and assigns them to the fields r, g, and b. The three-getter methods are used to retrieve the individual RGB values.
Gopi Gorantala Newsletter
Join the newsletter to receive the latest updates in your inbox.