Java Comments
Comments are a way to write notes for our code snippets, and a way to explain how the function/class works.
Table of Contents
In general, comments are notes that we write for our understanding. For example, If we read a book, we scribble on it, highlight some lines, write our own bullet points, etc. These are comments we write for our next reference. In the same way, comments are exactly the same in software.
Introduction
All programming languages, frameworks and libraries use comments. Comments are essential in trying to understand something which is written by a previous developer or a person who is working on a very big project.
In Java, comments are used to add explanatory notes or remarks to the code that are not executed by the compiler. They are ignored by the compiler and are only meant for human readers to understand the code more easily. There are three types of comments in Java:
- Single-line comments.
- Multi-line comments.
- Javadoc comments.
Comments are useful for documenting the code and making it more readable, especially for other programmers who may need to understand and modify the code in the future. Good commenting practices can also help prevent errors and make it easier to debug the code.
Sketch
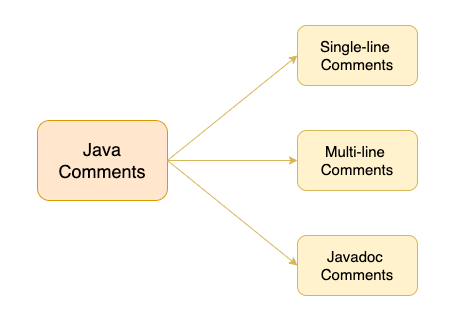
Single-line comments
Single-line comments are used to add comments or explanations to the code. These comments start with two forward slashes (//
) and extend to the end of the line.
Here's an example of a single-line comment in Java:
// This is a single line comment in Java
Anything written after //
on that line will be ignored by the Java compiler. Single-line comments are useful for adding quick notes or explanations to the code, as well as temporarily disabling a line of code during testing or debugging.
The following code snippet has multiple single-line comments in various places.
public class SingleLineComment {
public static void main(String[] args) {
int firstValue = 100; // integer variable with value `100`
int secondValue = 200; // integer variable with value `200`
// integer variable adding two values and storing the sum in `result` variable
int result = firstValue + secondValue;
// below line prints the `result` variable
System.out.println(result);
}
}
Above snippets output the following:
300
Multi-line comments
Multi-line comments are used to add comments or explanations that span multiple lines of code. These comments start with /*
and end with */
, and can span as many lines as needed.
Here's an example of a multi-line comment in Java:
/*
This is a multi-line comment
that spans multiple lines of code.
It is useful for adding detailed
notes or explanations to the code.
*/
Anything written between /*
and */
will be ignored by the Java compiler. Multi-line comments are particularly useful for adding detailed explanations to complex sections of code or for temporarily disabling a block of code during testing or debugging.
The following code snippet has a multi-line comment in various places.
public class MultiLineComment {
public static void main(String[] args) {
/*
We are declaring two variables `firstValue`, `secondValue` with `100`
and `200` values.
We declared another variable `result` and stored the sum in it.
Finally, we are printing the result on the console using `System.out.println()`.
*/
int firstValue = 100;
int secondValue = 200;
int result = firstValue + secondValue;
System.out.println(result);
}
}
Above snippets output the following:
300
Javadoc comments
Javadoc comments are a special type of comment used in Java to document classes, interfaces, methods, and fields. Javadoc comments start with /**
and end with */
, and can contain a variety of information, including the name and purpose of the class, interface, or method, as well as any parameters, return types, and exceptions.
/**
* Returns the sum of two integers.
*
* @param a the first integer
* @param b the second integer
* @return the sum of a and b
*/
public int add(int a, int b) {
return a + b;
}
Javadoc comments use a special syntax to indicate different types of information, such as @param
to indicate a method parameter or @return
to indicate the return value of a method. Javadoc comments are processed by the javadoc
tool, which can generate HTML documentation for the code.
Javadoc comments are an important part of creating well-documented and maintainable Java code and are often used in libraries and APIs to help users understand how to use the code.
Summation example
Let us refactor the above examples using Javadoc.
public class JavadocComment {
public static void main(String[] args) {
int firstValue = 100;
int secondValue = 200;
int result = add(firstValue, secondValue);
System.out.println(result);
}
/**
* Returns the sum of two integers.
*
* @param a the first integer
* @param b the second integer
* @return the sum of a and b
*/
public static int add(int a, int b) {
return a + b;
}
}
Using tags
Here's an example of a Javadoc comment for a class in Java that includes @author
and @date
tags:
/**
* This class represents a point in 2D space.
*
* @author Gopi Gorantala
* @date April 13, 2023
*/
public class Point {
// class implementation goes here
}
In this example, the Javadoc comment includes a brief description of the class, followed by the @author
tag, which indicates the name of the author of the code.
The comment also includes the @date
tag, which indicates the date the code was written or last modified. The date can be in any format that is easy to read, such as April 13, 2023
or 2023-04-13
.
Including these tags in Javadoc, comments can help other developers understand the purpose and history of the code, and can also help to ensure proper attribution for the work done.
Note:Does declaring class here feels intimidating? Don't worry, you will deep dive into each of the keywords you see inside the class declaration.
Gopi Gorantala Newsletter
Join the newsletter to receive the latest updates in your inbox.