How To Write Lambda Expressions
This lesson introduces the basic lambda expression structure with tips to write efficient code.
Table of Contents
First, let us look at the basic structure of lambda expression. This could help us understand writing lambdas easily.
Basic Structure
The basic structure of lambda expression is:
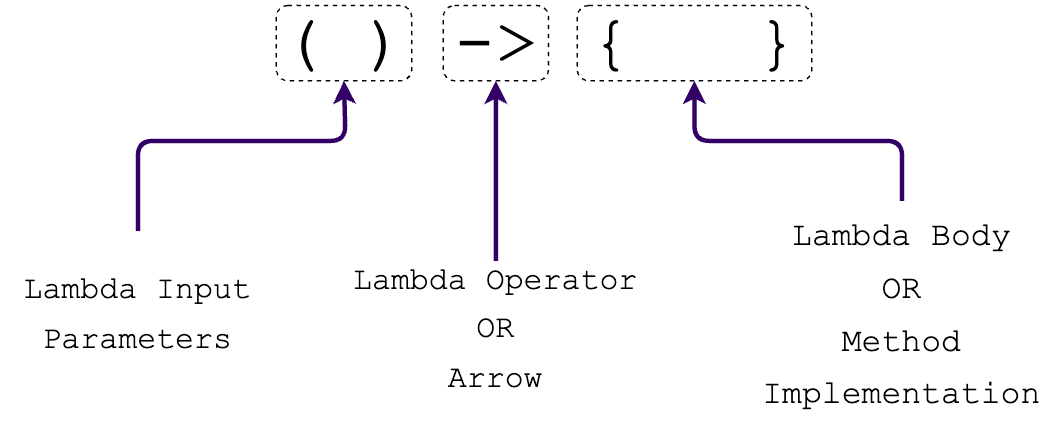
Basic steps
Java Lambda expression contains the following parts:
Parameters-list: This can be empty or contain one or more parameters, separated by commas. If there is only one parameter, you can omit the parentheses.
- Lambda operator: This separates the parameter list from the lambda expression's body or, in simple terms, connects the argument list with the lambda expression's body.
- Expression: Finish with the expression or statement(s) to be executed:
expression
or{ statements }
.
Example
Here is an example of a lambda expression that takes two integers as input and returns their sum:
(int a, int b) -> a + b
This lambda expression takes two integer parameters a
and b
and returns their sum. Note that the parameter types are specified in parentheses, and the return type is inferred based on the expression.
() -> System.out.println("Hello, world!")
This lambda expression has an empty parameter list, and the body contains a single statement to print a message to the console.
Lambda expressions can be assigned to functional interfaces or used directly as arguments to methods. For example, to use the above lambda expression with the Runnable
functional interface, you can write:
Runnable helloWorld = () -> System.out.println("Hello, world!");
This creates a Runnable
object that executes the lambda expression when its run
method is called.
Tips when writing lambda expressions
Parameter lists sometimes have zero or more parameters.
There are rules to follow when writing a lambda expression. We don’t need to follow all, but to improve code quality we have to follow these tips when writing a lambda expression.
- When there are no parameters, we should explicitly give
()
.
If there is a single parameter, we can ignore the parenthesis below. Both syntaxes are valid.
(message) -> {}
message -> {}
- When the body is a single line, we can exclude the curly braces
{
,}
andreturn
. The following example explains it.
// Both of the below expressions are valid.
// 01: Lambda expression printing Hello on console
() -> {
System.out.println("Hello");
}
// 02: Lambda expression printing Hello on console
() -> System.out.println("Hello");
- Java understands the underlined functional interface when running through the lambda expression. Because of this, we don’t need to have the parameter typed inside the parameters list.
// All of the below syntaxes print same output - Hello, fullName
(String fullName) -> {
System.out.println("Hello, " + fullName);
}
// Above can be refactored as follows.
(fullName) -> {
System.out.println("Hello, " + fullName);
}
// further more, we can replace it with
fullName -> System.out.println("Hello, " + fullName);
Gopi Gorantala Newsletter
Join the newsletter to receive the latest updates in your inbox.