Bit Manipulation
Introduction to Bit Manipulation
In order to ace coding interviews for tech companies, it is necessary to understand Bitwise operators, which are usually fast compared to arithmetic and other math operations.
Switch Sign of a Number
We make use of bit manipulation to solve this problem. using a NOT operator.
Subsets Or Powerset
In subsets or powerset problem, we need to write a program that finds all possible subsets (the power set) of a given input. The solution set must not contain duplicate elements.
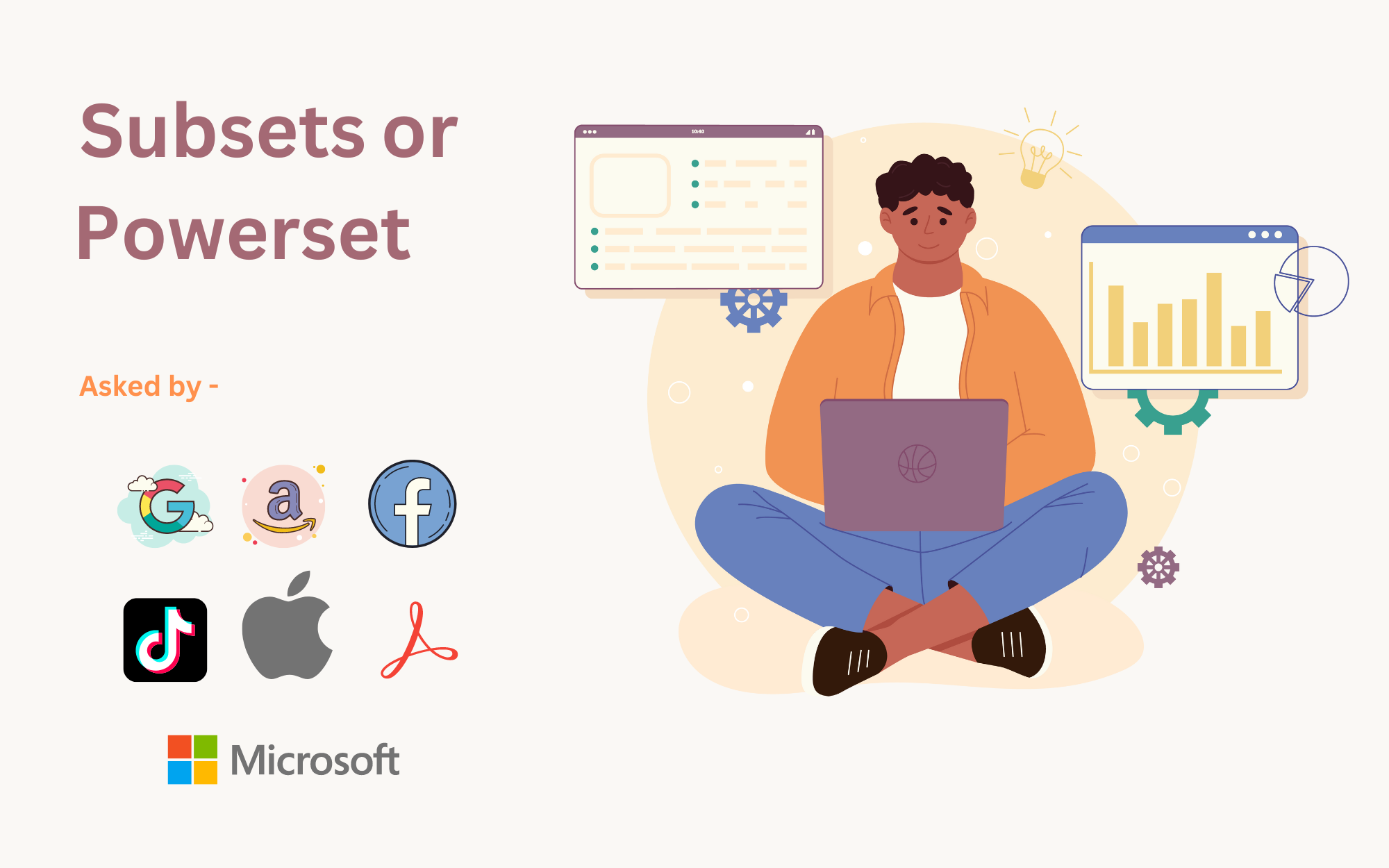
Challenge 2: Check If a Given Number is Even/Odd
This is an example of another Bitwise question. Think of the rightmost bit of a number and think of some logic that could solve this.
Counting Bits II
This is an extension to the counting set bits problem, counting the number of set bits or 1's present in a number. In here we see solutions in Java, JavaScript, Cpp, and TypeScript.
Challenge 1: Count Set Bits Or Number of 1 bit's
This is a traditional problem counting the number of set bits or 1's present in a number. Let’s see how we can count the set bits using the AND operator.
Bitwise AND, Computations, and Examples
A Bitwise AND is a binary operation that takes two equal-length binary representations and performs the logical AND operation on each pair of the corresponding bits. This is equivalent to multiplying them.
Introduction to Bitwise AND operator
Bitwise AND is the most commonly used logical Bitwise operator. It is represented by a sign (&). AND operator is the same as the AND gate we studied in the chapter on digital electronics.
Convert Decimal Numbers to Binary Numbers
We use the modulo and division method, and we calculate the binary representation of a decimal number. Let’s represent the binary by considering the remainder column from bottom to top.
Count the Number of Digits in an Integer
This is an example problem for counting digits in an integer. Solving this problem helps you find the place values and how they are represented in the decimal number system. Let’s see some approaches we can take to solve this algorithmic problem. Approach