NoSuchBeanDefinitionException: The Most Common Spring Bean Error
Learn how to fix NoSuchBeanDefinitionException in Spring Boot with clear examples and best practices for dependency injection, package scanning, and bean registration.
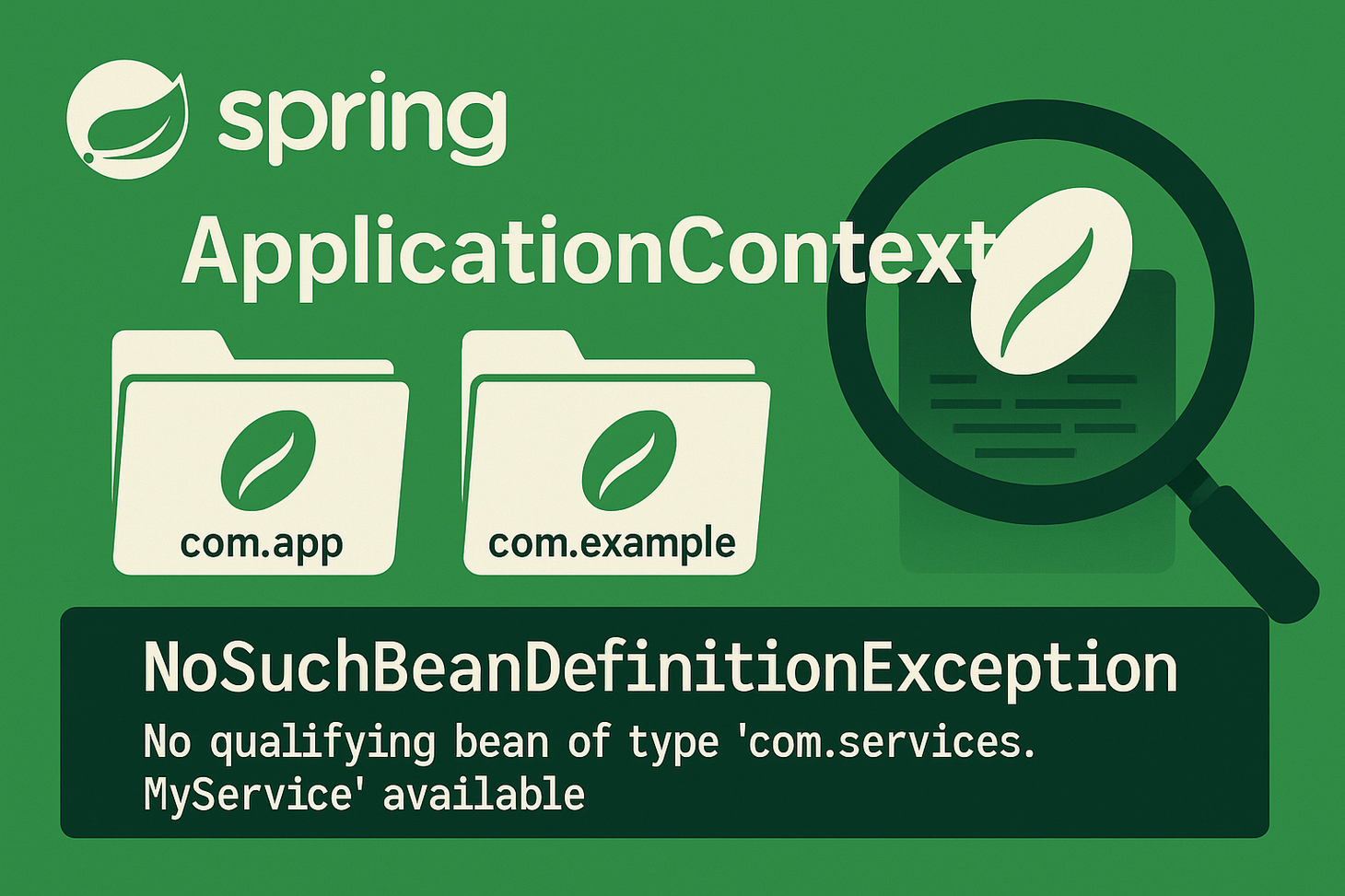
Table of Contents
When working with Spring’s dependency injection, one of the errors Java developers encounter most often is the dreaded:
***************************
APPLICATION FAILED TO START
***************************
Description:
Parameter 0 of constructor in com.example.MyService required a bean of type
'com.example.MyRepository' that could not be found.
Action:
Consider defining a bean of type 'com.example.MyRepository' in your configuration.
Or more simply:
org.springframework.beans.factory.NoSuchBeanDefinitionException:
No qualifying bean of type 'com.example.MyService' available
This exception indicates that Spring’s application context was asked for a bean it doesn’t know how to create or hasn’t been instructed to manage. Below, we’ll explore the root causes, illustrate common scenarios, and share best practices to prevent and fix this error.
What Is NoSuchBeanDefinitionException?
Spring manages objects—known as beans—in its ApplicationContext. When you ask Spring to inject or retrieve a bean of a particular type or name, it looks through its registry of managed beans. If it can’t find a matching bean definition, it throws NoSuchBeanDefinitionException
.
Key points:
- Type-based lookup: Asking for a bean by class or interface.
- Name-based lookup: Asking for a bean by its ID or alias.
- The exception message tells you exactly which type or name was missing.
Typical Causes
Missing Stereotype Annotation
@Service
public class MyService {
// ...
}
If you forget @Service
(or @Component
/ @Repository
) on MyService
, Spring won’t scan and register it.
Incorrect Package Scanning
By default, @SpringBootApplication
scans its own package and subpackages. If your service resides outside those packages, Spring won’t find it:
@SpringBootApplication(scanBasePackages = {"com.app", "com.example.services"})
Bean Defined in Configuration but Not Registered
Defining a @Bean
in a @Configuration
class that isn’t itself picked up:
@Configuration
public class MyConfig {
@Bean
public MyService myService() { … }
}
Ensure MyConfig
is in a scanned package or explicitly imported via @Import(MyConfig.class)
.
Multiple Bean Candidates Without Qualifier
If you define two beans of the same type:
@Component class FooService implements ServiceInterface { … }
@Component class BarService implements ServiceInterface { … }
Asking for ServiceInterface
injection yields:
NoUniqueBeanDefinitionException: expected single bean but found 2
This is related but highlights scanning issues. You can solve it with @Primary
or @Qualifier
.
Diagnosing the Error
- Read the Exception Message: It names the missing type or bean ID. That’s your starting point.
- Check Annotations: Ensure your class has
@Component
,@Service
,@Repository
, or@Controller
. For factory methods, ensure@Configuration
and@Bean
. - Verify Package Structure: The package of your main application or your explicit
@ComponentScan
must cover all components. - Inspect Profiles: Beans can be conditional on Spring profiles via
@Profile
. Make sure the active profile matches. - Review Configuration Imports: If you split configs, ensure that all are imported or scanned.
Example: Fixing a Missing Bean
Here’s a minimal example that fails:
// src/main/java/com/app/Application.java
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
// src/main/java/com/services/MyService.java
@Service
public class MyService { /* … */ }
// src/main/java/com/app/Runner.java
@Component
public class Runner implements CommandLineRunner {
private final MyService myService;
public Runner(MyService myService) { this.myService = myService; }
public void run(String... args) { /* … */ }
}
Error:
NoSuchBeanDefinitionException: No qualifying bean of type 'com.services.MyService'
Cause: MyService
is in com.services
, but the application class is in com.app
. Spring only scans com.app
and subpackages.
Solution: Move Application
to com
root, or add scanBasePackages
:
@SpringBootApplication(scanBasePackages = "com")
public class Application { … }
Best Practices to Avoid Bean Definition Issues
- Keep a Flat Package Structure: Place your main class at the root of your package hierarchy (e.g.,
com.example
). All subpackages will be scanned automatically. - Use Consistent Stereotypes: Always annotate beans with the proper stereotype (
@Service
,@Repository
, etc.), even if you think you’ll register them manually later. - Leverage Auto-Configuration in Spring Boot: When possible, rely on Spring Boot’s opinionated configuration. Custom
@Configuration
classes should sit alongside your main class. - Document Conditional Beans: If beans are profile-specific or conditional (
@ConditionalOnMissingBean
,@Profile
), clearly document or centralize those configs. - Validate on Startup: Spring’s
ApplicationContext
fails fast on startup when a required bean is missing. Treat startup failures as critical and resolve them immediately.
Finally, NoSuchBeanDefinitionException
is Spring’s way of saying, “I don’t know how to give you that bean.” In most cases, it comes down to annotation or package-scanning oversight. You can eliminate this error from your development workflow by structuring your project with a clear package hierarchy, consistently using stereotypes, and understanding how Spring Boot scans for beans.
Understanding the mechanics behind Spring’s bean registration and scanning process is key to rapid troubleshooting—and, ultimately, to writing more robust, maintainable Spring applications.
Gopi Gorantala Newsletter
Join the newsletter to receive the latest updates in your inbox.