What is the JVM? With Sketches and Examples
Table of Contents
Short answer
JVM stands for Java Virtual Machine.
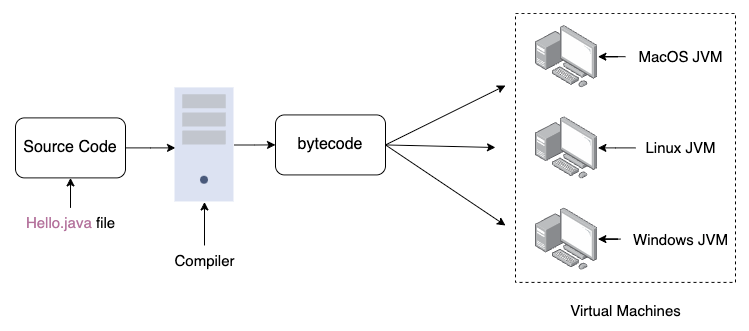
JVM is a virtual machine( a piece of software) that executes Java bytecode. It provides a runtime environment for executing Java applications. The above sketch explains how the same bytecode is run across multiple virtual machines on different operating systems.
If someone talks about the HotSpot, that's the name of the open source JVM developed by developers ar OpenJDK.
JVM includes features, such as memory management, an execution engine, a garbage collector, and a security manager. The JVM is the core component of the JRE and is responsible for executing Java applications.
More detailed answer
So, where does the JVM reside? How is the JDK bundled? Let us see a simple sketch that explains what is JDK, how it is bundled, and where JVM exists. This gives you clarity on how JDK-JRE-JVM is connected.
JVM Sketch
JDK sketch including all pieces of software. The only important ones are shown here:
JDK (Java Development Kit)
|
+--- JRE (Java Runtime Environment)
|
+--- JVM (Java Virtual Machine)
|
+--- Java Class Libraries
| |
| +--- java.lang Package
| |
| +--- java.io Package
| |
| +--- java.util Package
| |
| +--- ...
|
+--- Java Standard Extensions
|
+--- JavaFX
|
+--- Java Accessibility
|
+--- Java Cryptography Extension (JCE)
|
+--- ...
JVM is a part of JRE (Java Runtime Environment) and is used to convert the bytecode into machine-level language. This machine is responsible for allocating memory.
JDK is a software development kit provided by Oracle Corporation for developing Java applications. It includes:
- Java Runtime Environment (JRE),
- an interpreter/loader (Java),
- a compiler (javac),
- an archiver (jar),
- a documentation generator (Javadoc),
- and other tools needed in Java development.
In summary, the JDK includes everything necessary to develop and run Java applications, the JRE includes everything necessary to run Java applications, and the JVM is the virtual machine that executes Java bytecode and provides the runtime environment for running Java applications.
JVM memory areas
JVM divides the memory a Java application uses into several distinct areas, each serving a specific purpose in managing and executing Java programs.
These memory areas collectively constitute the JVM memory management subsystem and help efficiently organize memory allocation and deallocation.
The primary memory areas in the JVM are as follows:
- Heap memory
- Memory area (non-heap memory)
- Stack memory
- PC register
- Native method stack
Heap Memory
- Heap memory is the largest memory area in the JVM, where objects are dynamically allocated at runtime.
- All Java objects created by the application are stored in the heap.
Method area (non-heap memory)
- The method area is a non-heap memory area that stores class-level information, such as bytecode, method data, field data, and constant pool.
- Each loaded class has its representation in the method area.
- It is also known as the Permanent Generation (PermGen) in older JVM versions (Java 7 and earlier). In newer JVM versions (Java 8 and later), the PermGen space has been replaced by the Metaspace, a native memory area.
Java Stack Memory
- Each thread in a Java application has its own private Java stack memory.
- The Java stack stores method invocations, local variables, and partial results for each thread.
- It is used for method calls and method return tracking.
- The size of the Java stack is usually fixed per thread and is relatively small compared to the heap.
PC Register (Program Counter)
- The PC register holds the current execution address of the JVM instructions for each thread.
- It is used to keep track of the current instruction being executed by the thread.
Native Method Stack
- The native method stack stores native method calls and data for each thread.
- It is used to support native methods, which are methods written in languages other than Java and integrated into the Java application through the Java Native Interface (JNI).
The management of these memory areas is an essential aspect of the JVM's responsibilities. The garbage collector performs memory management by automatically reclaiming memory occupied by objects that are no longer in use, preventing memory leaks and ensuring efficient memory utilization. The JVM's memory management subsystem is designed to optimize performance, reduce overhead, and provide reliable memory management for Java applications.
Garbage collection
GC is only about memory. Besides memory, other resources need cleaning up. If you open a file, you need to remember to close that file. The JVM won't help you close files that you leave open. So if I write code that opens a file or a network socket or another external resource, I should also write code that releases that resource when I'm done with it.
The Garbage Collection in the JVM is a system that tracks all references and gets rid of objects only when they become unreachable. This process takes time, and it's unpredictable. You never know for sure when the garbage collector is going to kick in and clean up memory. That's why Java isn't an ideal language for strict runtime computations.
On the other hand, Java can't just delete this object when the reference is out of scope because there might be other references to the same object. There might be aliasing, so when is this object destroyed? That's why some languages say it's your business programmer.
You must keep track of your objects and know when to destroy them. Because you know they can't be reached anymore. And when you do, you call it destructor explicitly; if you get it wrong, that's one of the worst possible bugs. You are releasing memory on something that is still in use.
Luckily, we don't need to do that in Java because a Java program runs in a JVM, a virtual machine, and the JVM can track the references for us. If there is no reference pointing to an object, JVM will say this object is unreachable now and release its memory automatically. This process is called Garbage collection.
Gopi Gorantala Newsletter
Join the newsletter to receive the latest updates in your inbox.