How can I remove a specific item from an array in JavaScript?
Removing a specific item from an array is the most use case a developer runs into. You will learn more than 7 ways to achieve this.
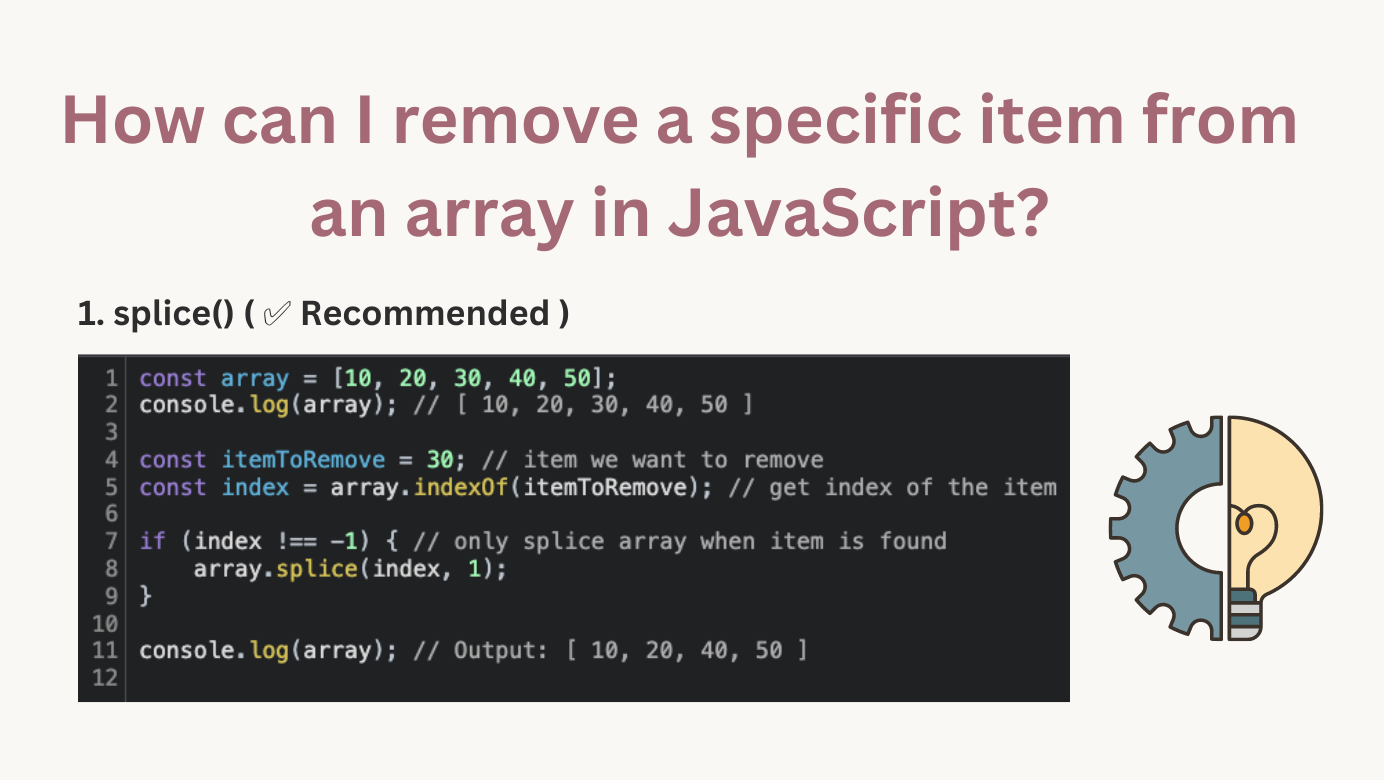
Table of Contents
Introduction
The most common use case a Javascript or frontend developer runs into is "removing an item from an array."
In JavaScript, removing an item from an array can be done in several ways.
🤑 Partner Discounts
- 20% off on Educative.io + 20% Holiday Season Promo
- ZeroToMastery 30% Flat Discount Code: PPP_30
- Get 50% Off From Skillshare
- Coursera Flat 70% off on selected courses
- Up to 20% discount on DataCamp (Limited Offer)
- Memrise: 28% discount on lifetime access (Join 65 million learners)
- Udacity: Up to 70% OFF on Udacity's nanodegree
- Flat 50% off on Linux Foundation boot camps, 30% on bundles, and certifications.
How to replicate this?
Let us assume we have some items in the array as follows:
const array = [10, 20, 30, 40, 50];
console.log(array); // [10, 20, 30, 40, 50]
You can run this snippet directly on google chrome developer tools under the sources tab, create a snippet, and you are good to run JavaScript snippets directly on the browser.
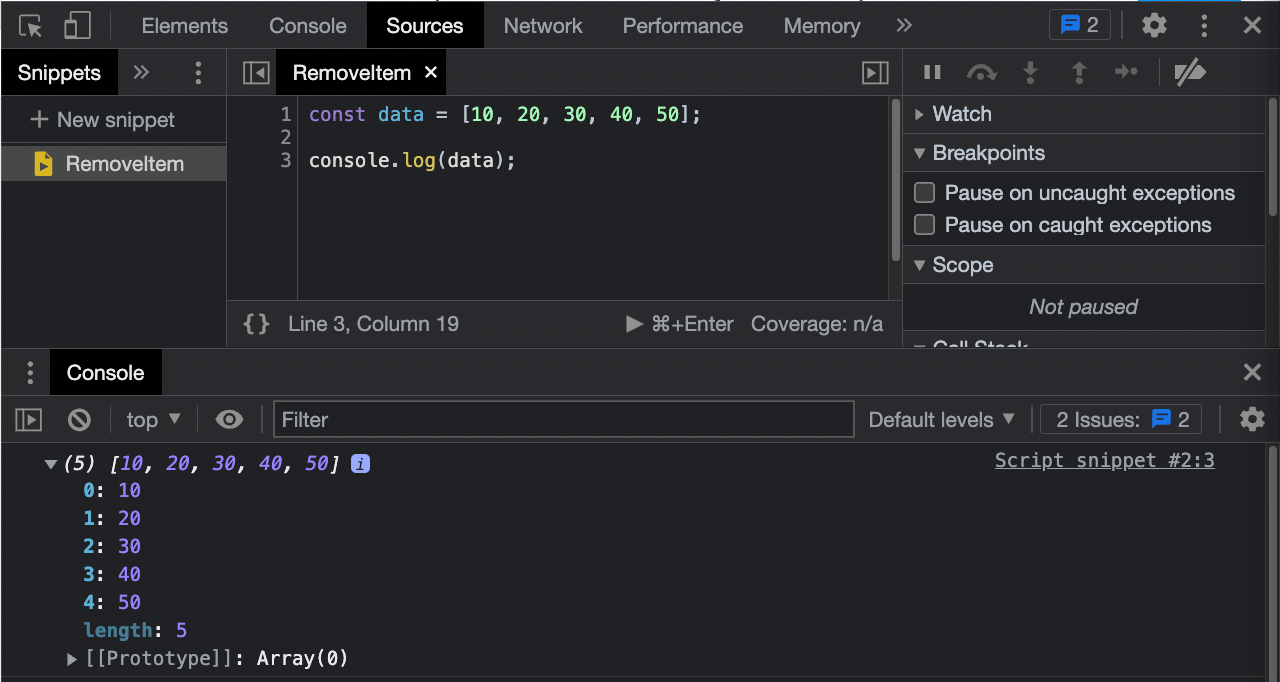
For example, to remove an element at the index 2
, which is the item 30
. We must write some logic to remove/delete the element from the array. Following are various ways we can remove an element from an array.
How can we achieve this?
There are different ways to remove a specific item from an array in JavaScript. Let us take a look at each of them.
- using
splice()
. - using
filter()
. - Using a traditional loop.
- Using
slice()
with spread operator - Using
indexOf()
andslice()
methods - Using
reduce()
- Using
splice()
andlastIndexOf()
Different ways to remove an item from the array
Using splice() ( ✅ Recommended )
The splice()
method is a built-in function in JavaScript that allows you to modify an array by adding, removing, or replacing elements. It can be used to change the contents of an array by removing or replacing existing elements or by adding new elements in their place.
The splice()
method operates directly on the array and returns the modified array.
Approach #1
The following array
variable holds an array of 5
elements. Let us remove an element at the index 2
.
const array = [10, 20, 30, 40, 50];
console.log(array); // [ 10, 20, 30, 40, 50 ]
// remove an element at index `2`
const indexToRemove = 2; // index of item to remove
array.splice(indexToRemove, 1);
console.log(array); // Output: [ 10, 20, 40, 50 ]
The splice()
method takes two arguments: the index of the item to remove and the number of items to remove. In this example, we remove one item from the index 2
.
Approach #2
What if we want to delete an item and don't know where the element is located in the array? How can we find the index of it?
JavaScript API has provided indexOf
a method to find the element's index in the array. Let us see the code in action.
const array = [10, 20, 30, 40, 50];
console.log(array); // [ 10, 20, 30, 40, 50 ]
const itemToRemove = 30; // item we want to remove
const index = array.indexOf(itemToRemove); // get index of the item
if (index !== -1) { // only splice array when item is found
array.splice(index, 1);
}
console.log(array); // Output: [ 10, 20, 40, 50 ]
Using filter()
The filter()
method creates a new array containing all the elements that pass a specific condition. You can use it to create a new array that excludes the item you want to remove. Here's an example:
let array = [10, 20, 30, 40, 50];
console.log(array); // [ 10, 20, 30, 40, 50 ]
const itemToRemove = 30; // item we want to remove
array = array.filter(function (item) {
return item !== itemToRemove;
});
console.log(array); // [ 10, 20, 40, 50 ]
Using a for-loop
You can iterate over the array and copy all elements except the one you want to remove into a new array.
Here's an example:
let array = [10, 20, 30, 40, 50];
console.log(array); // [ 10, 20, 30, 40, 50 ]
const itemToRemove = 30; // item we want to remove
const newArray = [];
for (let i = 0; i < array.length; i++) {
if (array[i] !== itemToRemove) {
newArray.push(array[i]);
}
}
console.log(newArray); // Output: [ 10, 20, 40, 50 ]
Using the slice() with the Spread operator
The slice()
method returns a shallow copy of a portion of an array into a new array. In this example, we create a new array by concatenating the original array's first portion up to the item's index to remove, with the second portion of the original array after the item's index to remove.
let array = [10, 20, 30, 40, 50];
console.log(array); // [ 10, 20, 30, 40, 50 ]
const indexToRemove = 2; // index to remove
const newArray = [...array.slice(0, indexToRemove), ...array.slice(indexToRemove + 1)];
console.log(newArray); // Output: [ 10, 20, 40, 50 ]
Using indexOf() and slice()
You can combine the indexOf()
method to find the index of the item and the slice()
method to create a new array excluding that item.
Here's an example:
let array = [10, 20, 30, 40, 50];
console.log(array); // [ 10, 20, 30, 40, 50 ]
var itemToRemove = 30; // item to remove
var index = array.indexOf(itemToRemove);
if (index !== -1) { // only slice array when item is found
array = array.slice(0, index).concat(array.slice(index + 1));
}
console.log(array); // Output: [ 10, 20, 40, 50 ]
Using reduce()
The reduce()
method can iterate over the array and accumulate the desired elements into a new array.
Here's an example:
let array = [10, 20, 30, 40, 50];
console.log(array); // [ 10, 20, 30, 40, 50 ]
const itemToRemove = 30; // item to remove
array = array.reduce(function (acc, curr) {
if (curr !== itemToRemove) {
acc.push(curr);
}
return acc;
}, []);
console.log(array); // Output: [ 10, 20, 40, 50 ]
Using splice() and lastIndexOf()
If you want to remove all occurrences of a specific item, you can combine splice()
with lastIndexOf()
to remove the items from the end of the array first.
Here's an example:
let array = [10, 20, 30, 40, 50];
console.log(array); // [ 10, 20, 30, 40, 50 ]
const itemToRemove = 30; // item to remove
let index = array.lastIndexOf(itemToRemove);
while (index !== -1) {
array.splice(index, 1);
index = array.lastIndexOf(itemToRemove);
}
console.log(array); // Output: [ 10, 20, 40, 50 ]
These are a few additional ways to remove a specific item from an array in JavaScript. Each approach may have its own advantages depending on your specific use case.
Conclusion
There are multiple ways to remove a specific item from an array in JavaScript. Whether you choose to use splice()
, filter()
, or iterate through the array, depending on your use case: selectively eliminate the item and update the array accordingly.
Your approach may depend on factors such as efficiency, code readability, or personal preference.
With these techniques in your toolkit, you can confidently manipulate arrays in JavaScript and remove specific elements as needed.
Recommended Courses:
The following courses help you learn true basics and advanced Javascript concepts.
- JavaScript in Detail: From Beginner to Advanced.
- Learn Object-Oriented Programming in JavaScript.
- Decode the Coding Interview in JavaScript: Real-World Examples.
- Meta Frontend Developer Professional Certificate (Coursera course by Meta)
- JavaScript: The Advanced Concepts.
- JavaScript Web Projects: 20 Projects to Build Your Portfolio.
📚 Top Recommended Books
- JavaScript: The Definitive Guide: Master the World's Most-Used Programming Language 7th Edition
- Eloquent JavaScript, 3rd Edition: A Modern Introduction to Programming 3rd Edition
- Learn JavaScript Quickly: A Complete Beginner’s Guide to Learning JavaScript, Even If You’re New to Programming (Crash Course With Hands-On Project)
- The Road to React: Your journey to master plain yet pragmatic React.js
Next up?
Read the following articles:
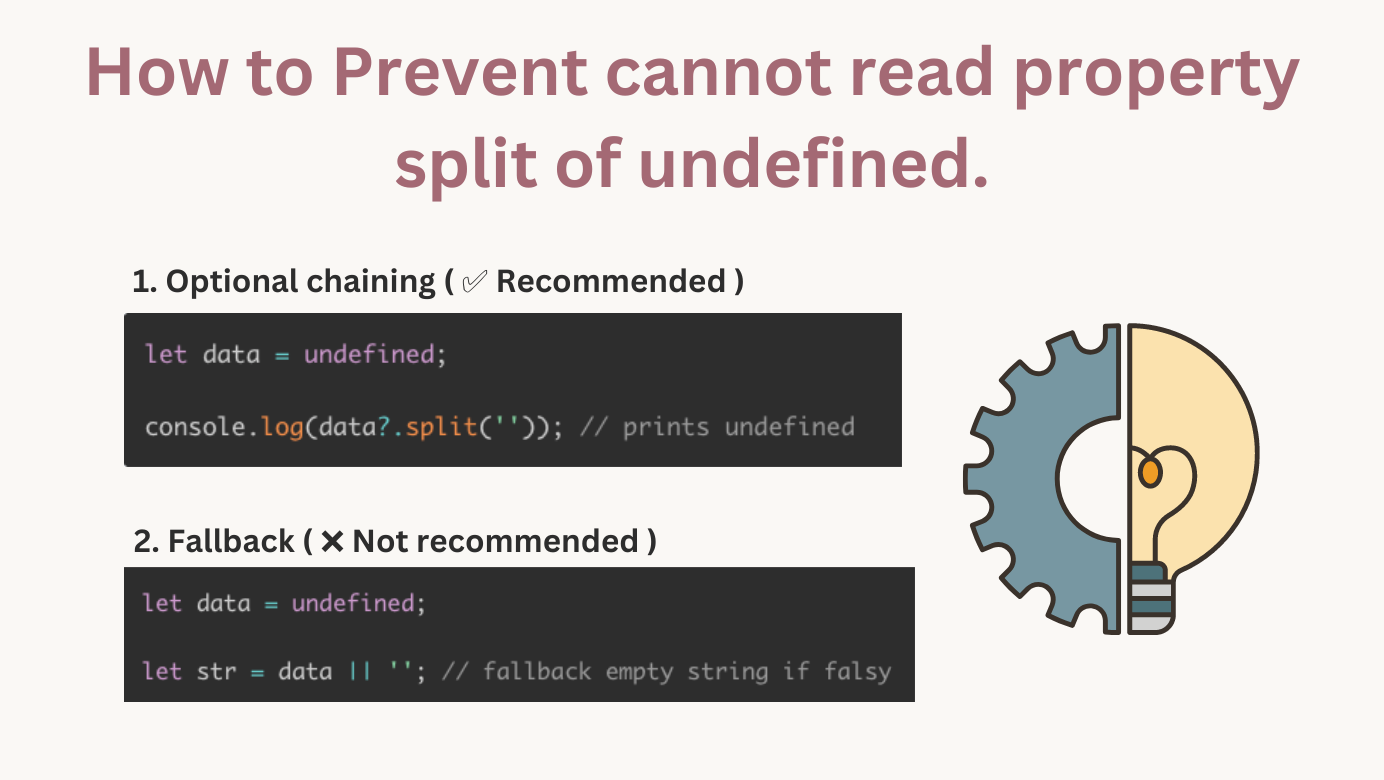
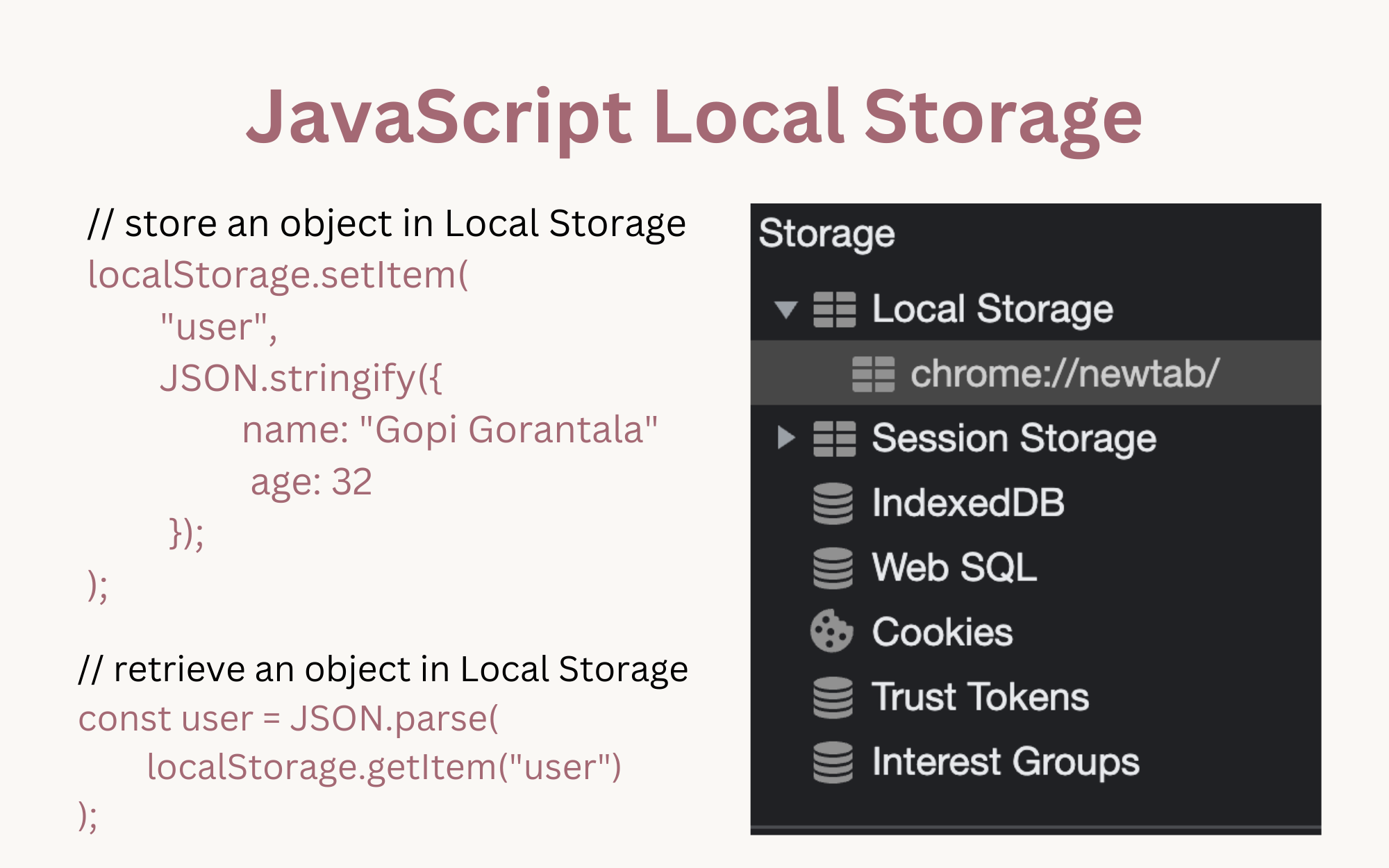
🤑 Partner Discounts
- 20% off on Educative.io + 20% Holiday Season Promo
- ZeroToMastery 30% Flat Discount Code: PPP_30
- Get 50% Off From Skillshare
- Coursera Flat 70% off on selected courses
- Up to 20% discount on DataCamp (Limited Offer)
- Memrise: 28% discount on lifetime access (Join 65 million learners)
- Udacity: Up to 70% OFF on Udacity's nanodegree
- Flat 50% off on Linux Foundation boot camps, 30% on bundles, and certifications.
Gopi Gorantala Newsletter
Join the newsletter to receive the latest updates in your inbox.