How to Prevent cannot read property split of undefined
The "cannot read property split of undefined" error occurs when we try to call the split() method on a variable that stores an undefined value.
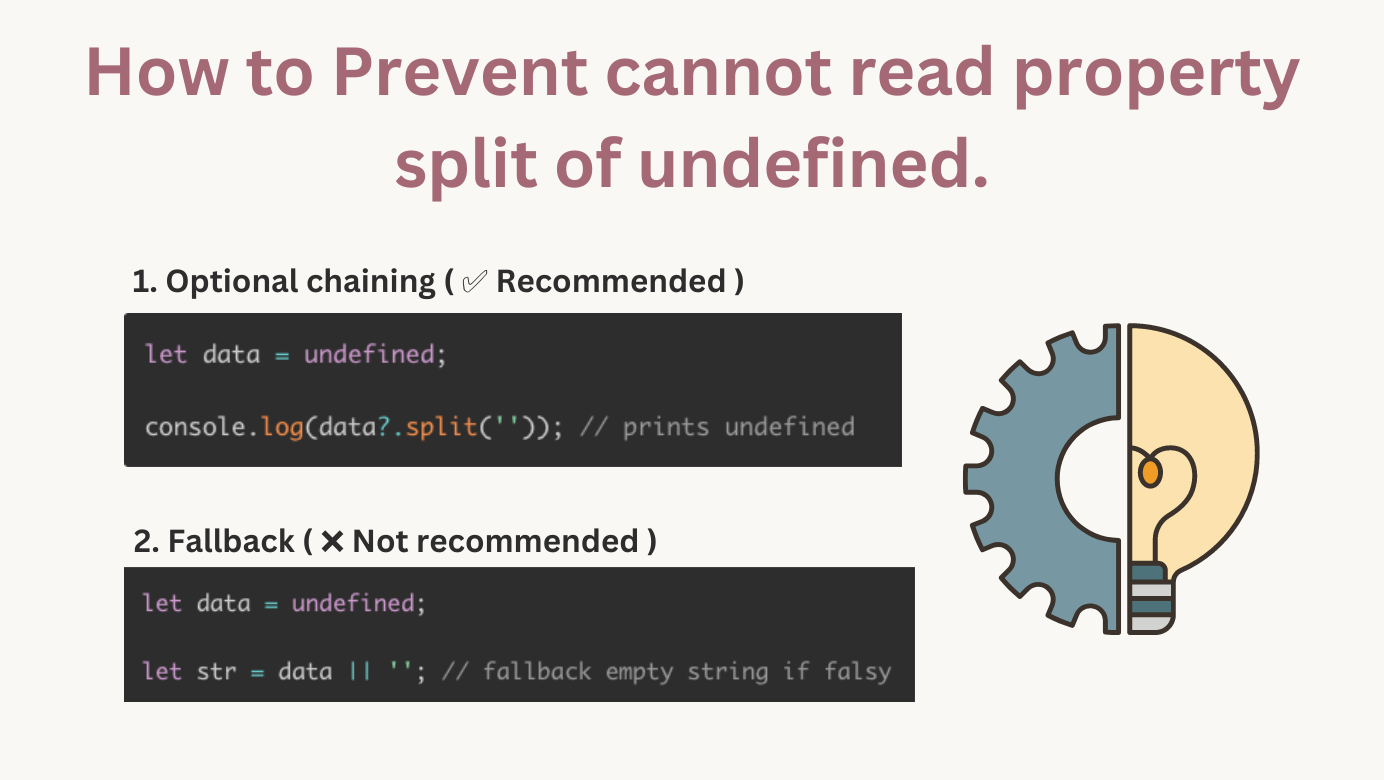
Table of Contents
Introduction
The "cannot read property split of undefined" error occurs when we try to call the split()
method on a variable that stores an undefined
value.
In JavaScript, a variable is said to be undefined
if it has been declared but not initialized. In simple words, when we do not assign any value to a variable, the JavaScript engine treats it as undefined
.
🤑 Partner Discounts
- 20% off on Educative.io + 20% Holiday Season Promo
- ZeroToMastery 30% Flat Discount Code: PPP_30
- Get 50% Off From Skillshare
- Coursera Flat 70% off on selected courses
- Up to 20% discount on DataCamp (Limited Offer)
- Memrise: 28% discount on lifetime access (Join 65 million learners)
- Udacity: Up to 70% OFF on Udacity's nanodegree
- Flat 50% off on Linux Foundation boot camps, 30% on bundles, and certifications.
How do we replicate this error?
Following are the two ways how JavaScript engine treats a variable as undefined.
- When we assign
undefined
to a variable.
let data = undefined;
console.log(data.split(','); // Uncaught TypeError: Cannot read properties of undefined (reading 'split')
2. When we declare a variable without assigning any value.
let data;
console.log(data.split(','); // Uncaught TypeError: Cannot read properties of undefined (reading 'split')
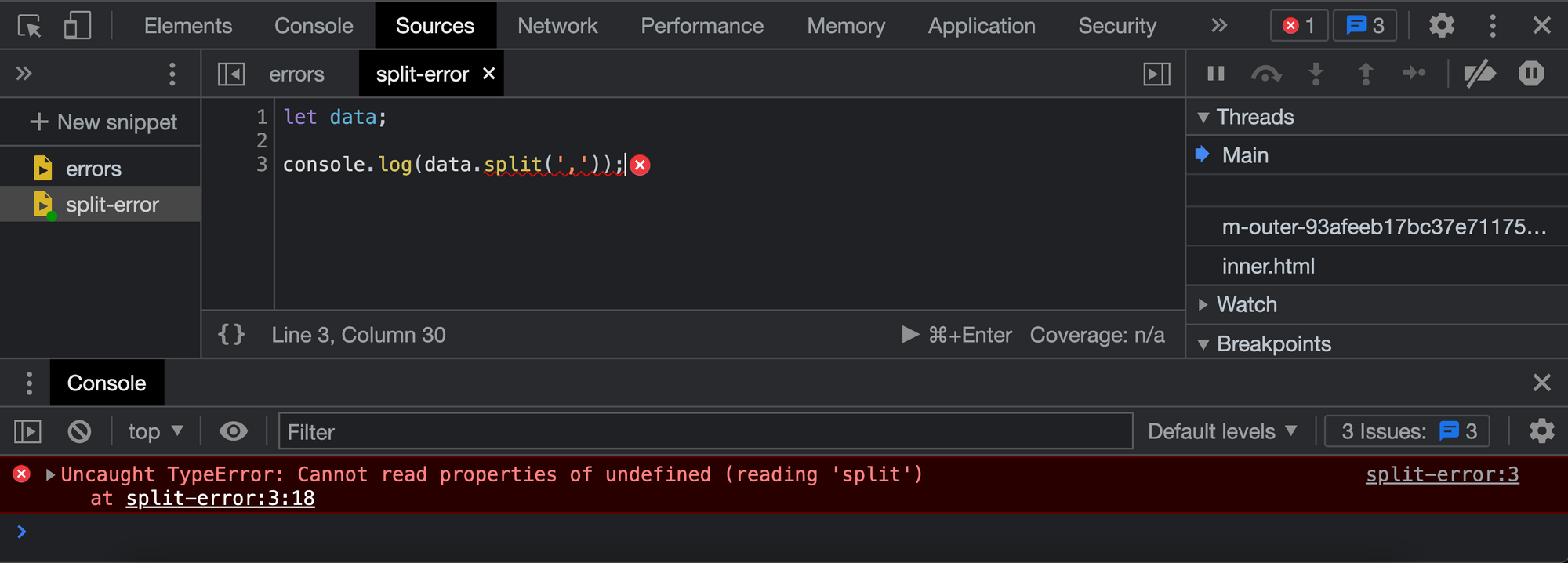
In both of the above snippets, we cannot call split()
method on a variable that has an undefined
value.
In this article, you will learn how these errors appear on the application and different methods to solve them. We will also see similar split
errors with null
object
, APIs etc.
The following courses help you learn true basics and advanced Javascript concepts.
- JavaScript in Detail: From Beginner to Advanced.
- Learn Object-Oriented Programming in JavaScript.
- Decode the Coding Interview in JavaScript: Real-World Examples.
- Meta Front-end Developer Professional Certificate (Coursera course by Meta)
- JavaScript: The Advanced Concepts.
- JavaScript Web projects: 20 Projects to Build Your Portfolio.
How to fix this error?
There are quite a few ways to solve this error
- Using
typeOf
- Equality check
- Optional chaining (✅ recommended)
- Fallback (❌ not recommended)
- Using
try/catch
typeof
keyword
A). We need to call the split
method only on strings. The following check will let us know if the variable we are dealing with is a string or any other type.
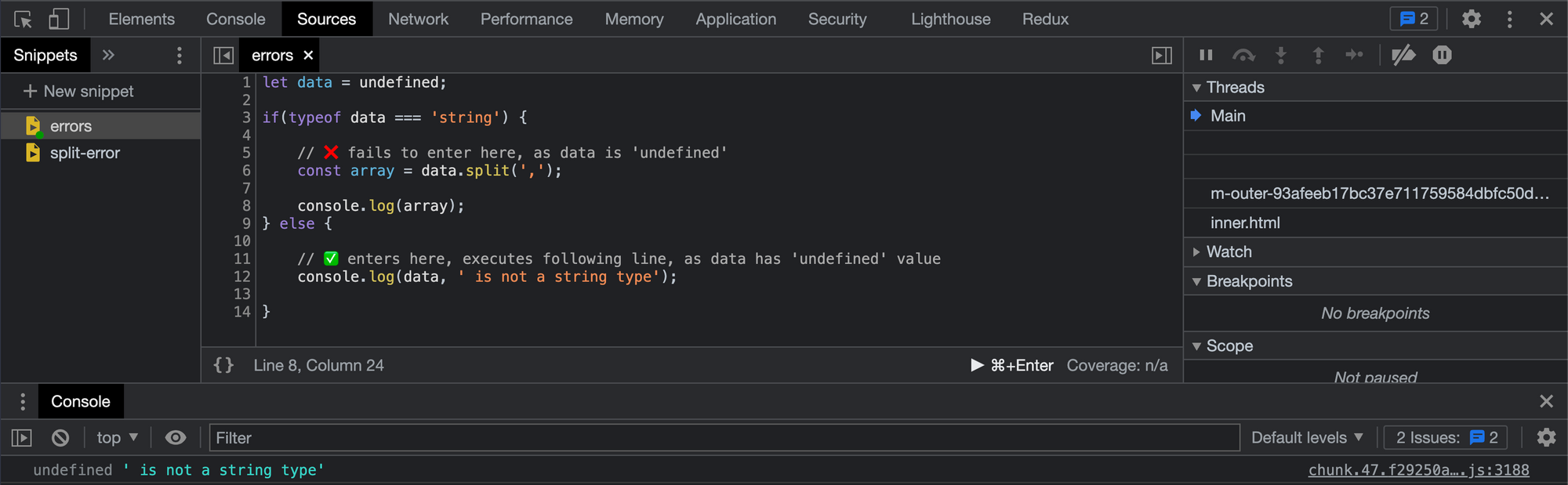
B). Alternatively, typeof
can also be used to determine whether a variable is of undefined
type.
let data;
if(typeof data !== 'undefined') {
// these statements execute
}
Equality check
We can use undefined
and the strict equality to determine whether a variable has a value.
let data; // this stores undefined
if(data !== undefined) {
// executes these statements
} else {
//these statements do not execute
}
Optional chaining ( ✅ Recommended )
let data = undefined;
console.log(data?.split('')); // prints undefined
The optional chaining operator ?.
will call the split()
method if the data
variable is not equal to undefined
or null
otherwise, it will return undefined, and no error is thrown.
Fallback ( ❌ Not recommended )
let data = undefined;
let str = data || ''; // fallback empty string if falsy
If data has undefined, then an empty string is stored in the variable data
.
try, catch
let data = undefined;
try {
// ❌ Fails this condition, as data is a undefined
const array = data.split(' ');
} catch (error) {
// ✅ executes this line, as data has undefined value
console.log('Something happened! 🤔', error);
}
// outputs
// Something happened! 🤔 TypeError: Cannot read properties of undefined (reading 'split')
We can use try and catch block, so when we call split()
on undefined
the catch block executes an application that won't be interrupted. Every day try/catch could be used to check for any issues with APIs.
How could this sort of situation happen?
This error occurs when you try to operate on a value variable. How can we stop this from happening?
There are a few other ways we, as a programmer, make a mistake and run into this error.
Querying APIs
When querying an API, we get the data and rarely do we run into an object property that possibly has an undefined
or null
value.
let data = {
val: undefined
};
console.log(data.val.split(',')); // Uncaught TypeError: Cannot read properties of undefined (reading 'split')
Trying to use a variable before assigning a value
In the following example, we call the split()
method before setting a value to it. Though we assigned a string value later, data
still holds undefined
value when we called split()
on it.
let data;
console.log(data.split(',')); // data still holds undefined
data = 'JavaScript,Java,C++'; // Uncaught TypeError: Cannot read properties of undefined (reading 'split')
Why does the split method only work for strings?
The split method happens to be a function on the string prototype. It only works on strings and always returns an array of string
types. To learn more about what split can do and how it can be done, here is the reference to the MDN doc - https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String/split
Why do you have to care about these errors?
One of many reasons to care about these errors is not to have any interruption when end users work on our software product.
Error handling should be the highest priority when building software to provide end users with an uninterrupted and seamless experience.
If you are working on a simple string or a massive object, you need proper error handling to ensure the application runs bug-free.
Things change and advance over time, technology included. Time doesn't spare software, and therefore bugs can appear.
Based on the end user's needs for our in-house software product or any application dependent on external APIs (let us say payment integrations, social sharing, or some other APIs etc.), error handling should be taken care of before consuming the data from them. This error handling helps gracefully handle hardware and software errors and helps execution resume when interrupted.
Important Note: No software is perfect, a zero bug development is a myth. Companies like Facebook, Netflix, Amazon, Dropbox, and other companies improve their software, they make improvements to meet the end users evolving expectations.
What is in-house product development?
Having everything developed in-house can reduce many bugs caused by external APIs due to dependencies.
In-house product development means everything is designed within the company and does not depend on any other external APIs that cause side effects. When we have everything built in-house, do you still need to care for the error handling? The answer is yes. No matter which object we pull, we first need to check if it is not null
or undefined
.
Real-Life Example: Airbnb
Airbnb is dependent on Google APIs to provide the exact latitude and longitude. So what if Google introduces some new properties and renames some existing ones?
It impacts Airbnb directly as there is a change in property names and some new properties added. Due to this, every software product should have error handling. In JavaScript, the best way to handle these is with the help of an optional chaining operator ?.
.
Real-Life Example: Toggle feature
Most of the top tech giants use "feature toggling". Feature toggling means they can turn on/off a feature from the product with a single global variable defined in the properties. If you want to enable or disable a feature on the product, it can quickly be done using this global variable.
Imagine a use case where a company wants to add multi-factor authentication to all its users on the platform. Let's say the variable twoFactorEnabled
is used for feature toggling. What if this variable is undefined
? The product thinks that this feature is disabled and will make sure nothing breaks and the application renders properly, but the multi-factor authentication feature is turned off or disabled.
Useful Tip: You can go to chrome dev tools, go to sources tab and add javascript code snippets, you can execute them directly on the browser instead having node installed to run plain JS snippets other ways.
Conclusion
The "cannot read property 'split' of undefined" error occurs when trying to call split()
method on a variable that stores an undefined
value.
To solve this error, make sure you call split()
method only on strings. The best way to check whether a variable is of string or other types, use the optional chaining discussed above.
Recommended Courses:
The following courses help you learn true basics and advanced Javascript concepts.
- JavaScript in Detail: From Beginner to Advanced.
- Learn Object-Oriented Programming in JavaScript.
- Decode the Coding Interview in JavaScript: Real-World Examples.
- Meta Front-end Developer Professional Certificate (Coursera course by Meta)
- JavaScript: The Advanced Concepts.
- JavaScript Web projects: 20 Projects to Build Your Portfolio.
Top Recommended Books
- JavaScript: The Definitive Guide: Master the World's Most-Used Programming Language 7th Edition
- Eloquent JavaScript, 3rd Edition: A Modern Introduction to Programming 3rd Edition
- Learn JavaScript Quickly: A Complete Beginner’s Guide to Learning JavaScript, Even If You’re New to Programming (Crash Course With Hands-On Project)
- The Road to React: Your journey to master plain yet pragmatic React.js
Gopi Gorantala Newsletter
Join the newsletter to receive the latest updates in your inbox.