A Complete Guide To JavaScript LocalStorage
localStorage is a property of the window object in JavaScript that allows you to store key/value pairs in a web browser. The data stored in localStorage persist even after the browser is closed, making it a useful tool for saving user data on the client side.
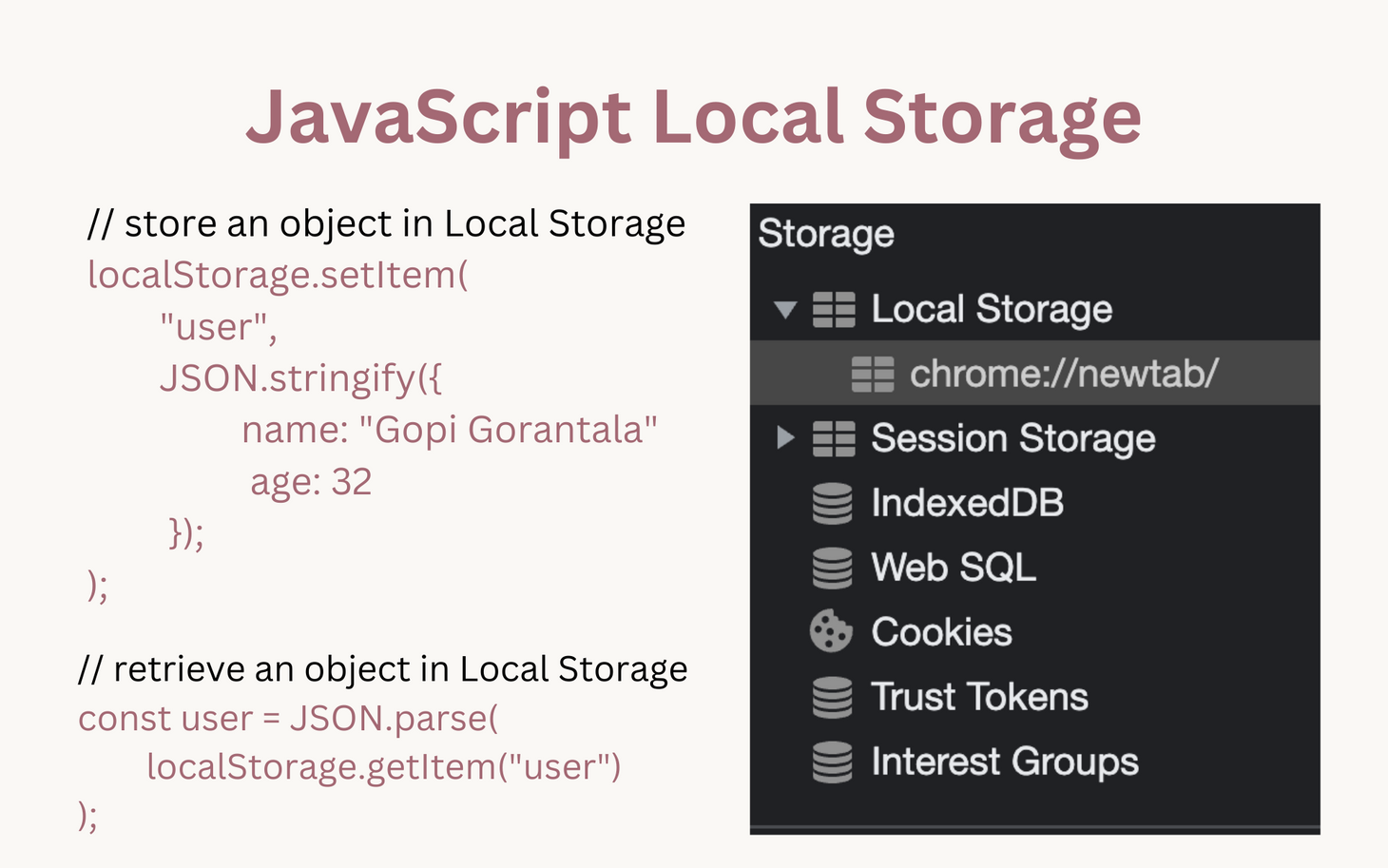
Table of Contents
In this article, you will learn everything you need to know about localStorage
.
What is Local Storage, and why do we need them?
localStorage
, is a property of the window
object in JavaScript that allows you to store key/value pairs in a web browser.
The data stored in localStorage
persist even after the browser is closed, making it a useful tool for saving user data on the client side. In simple words,localStorage
it is similar to sessionStorage
, except that localStorage
data has no expiration time, while sessionStorage
data gets cleared when the page session ends — when the page is closed.
The main features of localStorage
are:
- Shared between all tabs and windows from the same origin.
- The data does not expire. It remains after the browser restart and even OS reboots.
The localStorage
object has two main methods:
setItem(key, value)
- Used to store a key/value pair in localStorage.getItem(key)
- Used to retrieve the value associated with the specified key from localStorage.
Note: localStorage
has a limited size (typically around 5MB) and the stored data is only accessible by the same domain that stored it.
Local Storage API
localStorage
is a part of the Web Storage API and is widely supported by modern browsers.
Here's an example of how to use the JavaScript localStorage
API to store and retrieve data:
// Store data in localStorage
localStorage.setItem("key", "value");
// Retrieve data from localStorage
var data = localStorage.getItem("key");
console.log(data); // Output: "value"
// Update data in localStorage
localStorage.setItem("key", "new value");
// Retrieve updated data from localStorage
var updatedData = localStorage.getItem("key");
console.log(updatedData); // Output: "new value"
// Remove data from localStorage
localStorage.removeItem("key");
// Check if data has been removed
var removedData = localStorage.getItem("key");
console.log(removedData); // Output: null
// Clear all data from localStorage
localStorage.clear();
Note that localStorage
can only store strings, so if you need to store complex data structures like objects or arrays, you'll need to serialize them to a string format before storing and deserializing them when retrieving them. A common way to do this is to use JSON.stringify
and JSON.parse
, respectively:
// Store an object in localStorage
localStorage.setItem("user", JSON.stringify({ name: "John Doe", age: 30 }));
// Retrieve the object from localStorage
var user = JSON.parse(localStorage.getItem("user"));
console.log(user); // Output: { name: "John Doe", age: 30 }
Where exactly is the data getting stored in the browser?
The data we put in using localStorage
is stored in browser developer tools or something local to only browsers.
Steps:
- Open Google Chrome or Brave browser.
- Right-click in the middle of the screen and click on "Inspect", Chrome/Brave developer tools are shown.
- Click on the "Application" tab.
- On the left sidebar, you will see objects such as 'Application', 'Storage', 'cache', etc.
In Storage
, image is shown below, you will see the following options. In particular, we focus on Local Storage, so let's not sidetrack others. You will find another interesting article on each of them in detail.
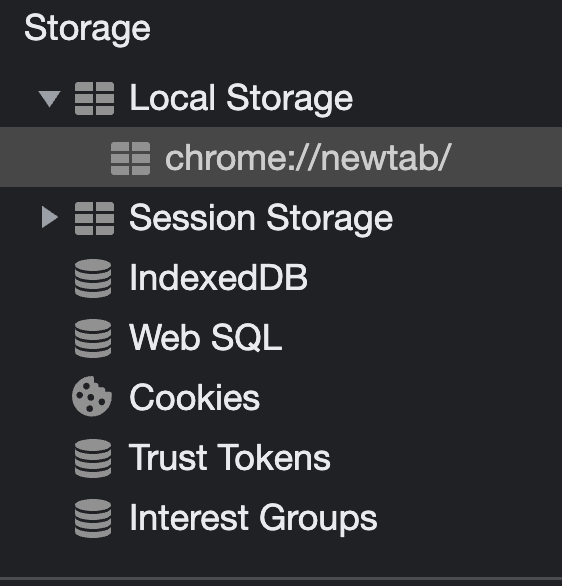
I have added an object and an entry in the localStorage
. Image is shown below:
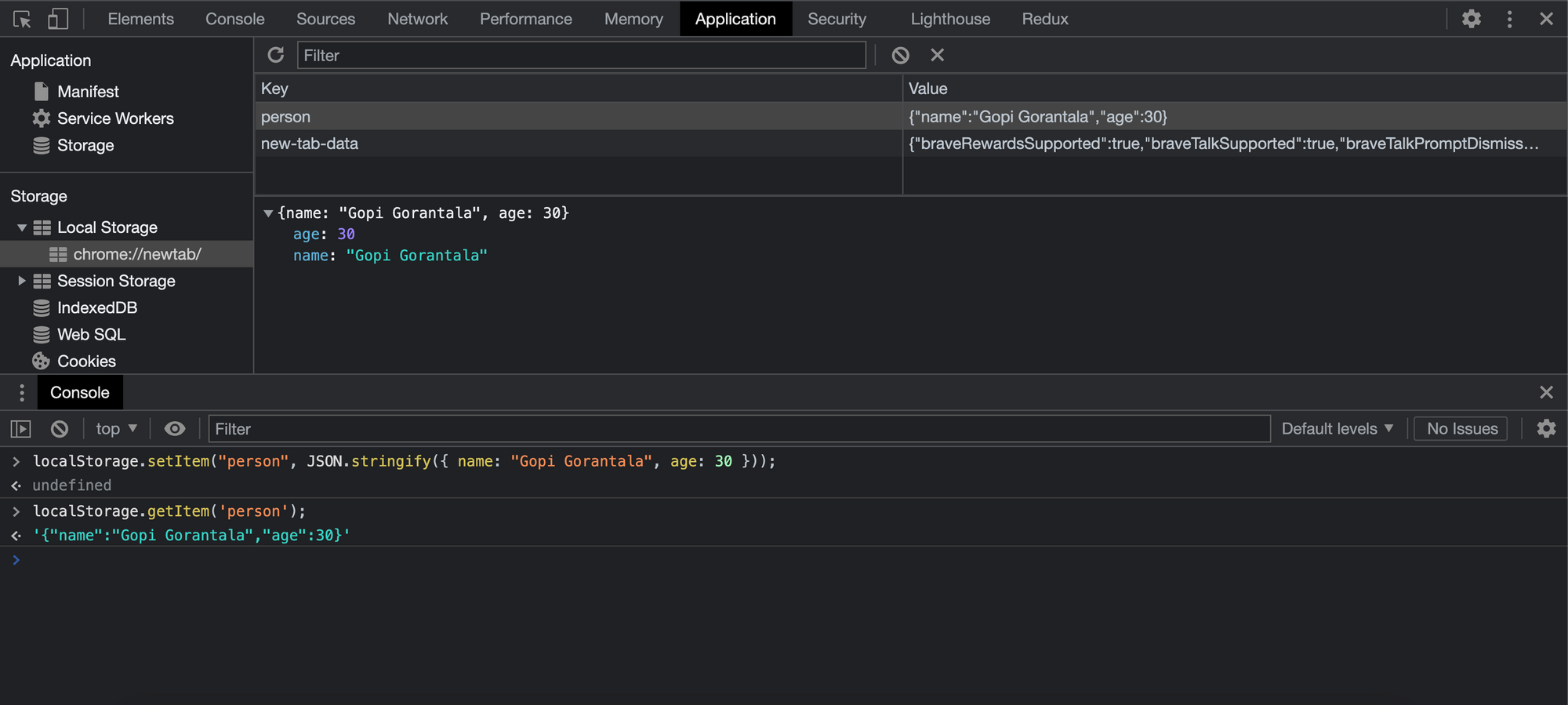
How to store objects?
Remember, everything in localStorage
is about strings. So we need to convert the object into a string using JSON.stringify(...);
Here's an example of how you can store an object in localStorage and then update it:
// Store an object in localStorage
var user = { name: "John Doe", age: 30 };
localStorage.setItem("user", JSON.stringify(user));
// Retrieve the object from localStorage
var retrievedUser = JSON.parse(localStorage.getItem("user"));
console.log(retrievedUser); // Output: { name: "John Doe", age: 30 }
// Update the object
retrievedUser.age = 31;
localStorage.setItem("user", JSON.stringify(retrievedUser));
// Retrieve the updated object
var updatedUser = JSON.parse(localStorage.getItem("user"));
console.log(updatedUser); // Output: { name: "John Doe", age: 31 }
In this example, the object user
is first converted to a JSON string using JSON.stringify
before being stored in localStorage using localStorage.setItem
. When retrieving the object from localStorage, it is then parsed back into a JavaScript object using JSON.parse
. This is necessary because localStorage only supports storing strings.
Write up a Service class for re-usability (A design pattern)
If you have been writing code for some time, then you already know that instead of duplicating localStorage
, we can write up a service that does everything for us in a single file, and we import it our react
, js
, angular
, and vue
applications on the fly.
I have written a class LocalStorageService
here as an example for your web app. Feel free to use it.
class LocalStorageService {
static add(key, value) {
try {
localStorage.setItem(key, JSON.stringify(value));
} catch (e) {
LogService.error({ error: JSON.stringify(e, Object.getOwnPropertyNames(e)), message: `Error when adding a key ${key} to localStorage` });
}
}
static get(key) {
try {
return JSON.parse(localStorage.getItem(key));
} catch (e) {
LogService.error({ error: JSON.stringify(e, Object.getOwnPropertyNames(e)), message: `Error when getting a key ${key} from localStorage` });
}
}
static remove(key) {
localStorage.removeItem(key);
}
}
export default LocalStorageService;
You can then use this class to store and retrieve data from localStorage in your application:
// Store an object in localStorage
LocalStorageService.setItem("user", { name: "John Doe", age: 30 });
// Retrieve the object from localStorage
var user = LocalStorageService.getItem("user");
console.log(user); // Output: { name: "John Doe", age: 30 }
// Update the object
user.age = 31;
LocalStorageService.setItem("user", user);
// Retrieve the updated object
var updatedUser = LocalStorageService.getItem("user");
console.log(updatedUser); // Output: { name: "John Doe", age: 31 }
Here are a few more examples of using the localStorage
API in JavaScript:
// Store an array of numbers
localStorage.setItem("numbers", JSON.stringify([1, 2, 3, 4, 5]));
// Retrieve the array from localStorage
var numbers = JSON.parse(localStorage.getItem("numbers"));
console.log(numbers); // Output: [1, 2, 3, 4, 5]
// Store a boolean value
localStorage.setItem("isLoggedIn", JSON.stringify(true));
// Retrieve the boolean value from localStorage
var isLoggedIn = JSON.parse(localStorage.getItem("isLoggedIn"));
console.log(isLoggedIn); // Output: true
// Store a user object with multiple properties
var user = {
name: "Jane Doe",
email: "jane@example.com",
age: 35,
isAdmin: false
};
localStorage.setItem("user", JSON.stringify(user));
// Retrieve the user object from localStorage
var storedUser = JSON.parse(localStorage.getItem("user"));
console.log(storedUser);
// Output:
// {
// name: "Jane Doe",
// email: "jane@example.com",
// age: 35,
// isAdmin: false
// }
Best practices
Here are a few tips for effectively using localStorage in JavaScript:
- Size Limitations: Keep in mind that localStorage has a limit of around 5 MB, so it's unsuitable for storing large amounts of data.
- Data Types: localStorage can only store strings, so you'll need to serialize objects before storing them and deserialize them when retrieving them.
- Security: localStorage data is stored on the client side and is accessible by any script on the same origin, so be careful what information you store. Do not store sensitive information such as passwords or financial data.
- Cross-Tab Synchronization: localStorage data is shared between tabs and windows of the same origin, so changes made in one tab will be reflected in all others.
- Expiration: localStorage data does not expire by default, so you'll need to manually manage it if you need data to be automatically removed after a certain time period.
- Debugging: It can be helpful to use browser developer tools to inspect
localStorage
data and monitor its usage in your web app. - Testing: When testing your web app, remember that different browsers have different implementations of
localStorage
and may behave differently.
Some important points about localStorage are:
- Data stored in localStorage is limited to the same origin (i.e. domain and port) that stored it.
- The data stored in localStorage is not automatically sent to the server with every HTTP request. It remains on the client side.
- The data stored in localStorage does not expire and remains until it is explicitly deleted, or the user clears their browser cache.
- localStorage is synchronous, meaning that any operation that reads or writes data to localStorage will block the JavaScript execution until it is complete.
- The data stored in localStorage is limited to strings, so you will need to convert any data you want to store to a string format using JSON.stringify and parse it back using JSON.parse when reading it.
- localStorage should be used with caution, as it is possible for malicious code to access and manipulate data stored in localStorage, so it should not be used to store sensitive information such as passwords or credit card numbers.
📚 Top Recommended Books
- JavaScript: The Definitive Guide: Master the World's Most-Used Programming Language 7th Edition
- Eloquent JavaScript, 3rd Edition: A Modern Introduction to Programming 3rd Edition
- Learn JavaScript Quickly: A Complete Beginner’s Guide to Learning JavaScript, Even If You’re New to Programming (Crash Course With Hands-On Project)
- The Road to React: Your journey to master plain yet pragmatic React.js
Conclusion
In conclusion, the localStorage
API is a useful tool for storing and retrieving data in JavaScript. It provides a simple way to persist data in the browser even after it is closed, making it ideal for maintaining user data across sessions.
When using localStorage
, it's important to remember that it can only store strings. Hence, complex data structures like objects and arrays must be serialized to a string format before storing and deserialized when retrieving. Overall, localStorage
is a powerful and easy-to-use feature of modern browsers that can greatly enhance the user experience of your web applications.
🤑 Partner Discounts
- 20% off on Educative.io + 20% Holiday Season Promo
- ZeroToMastery 30% Flat Discount Code: PPP_30
- Get 50% Off From Skillshare
- Coursera Flat 70% off on selected courses
- Up to 20% discount on DataCamp (Limited Offer)
- Memrise: 28% discount on lifetime access (Join 65 million learners)
- Udacity: Up to 70% OFF on Udacity's nanodegree
- Flat 50% off on Linux Foundation boot camps, 30% on bundles, and certifications.
Gopi Gorantala Newsletter
Join the newsletter to receive the latest updates in your inbox.