Coding Interview Questions
The practice you need to ace the coding interviews.
Power Of Two (Exercise Problem)
This is an exercise problem for your practice. Try to come up with an approach and solve it by yourself. Good Luck!
How To Construct An Array-Like Data Structure?
You will learn to implement a custom array-like data structure and basic array operations.
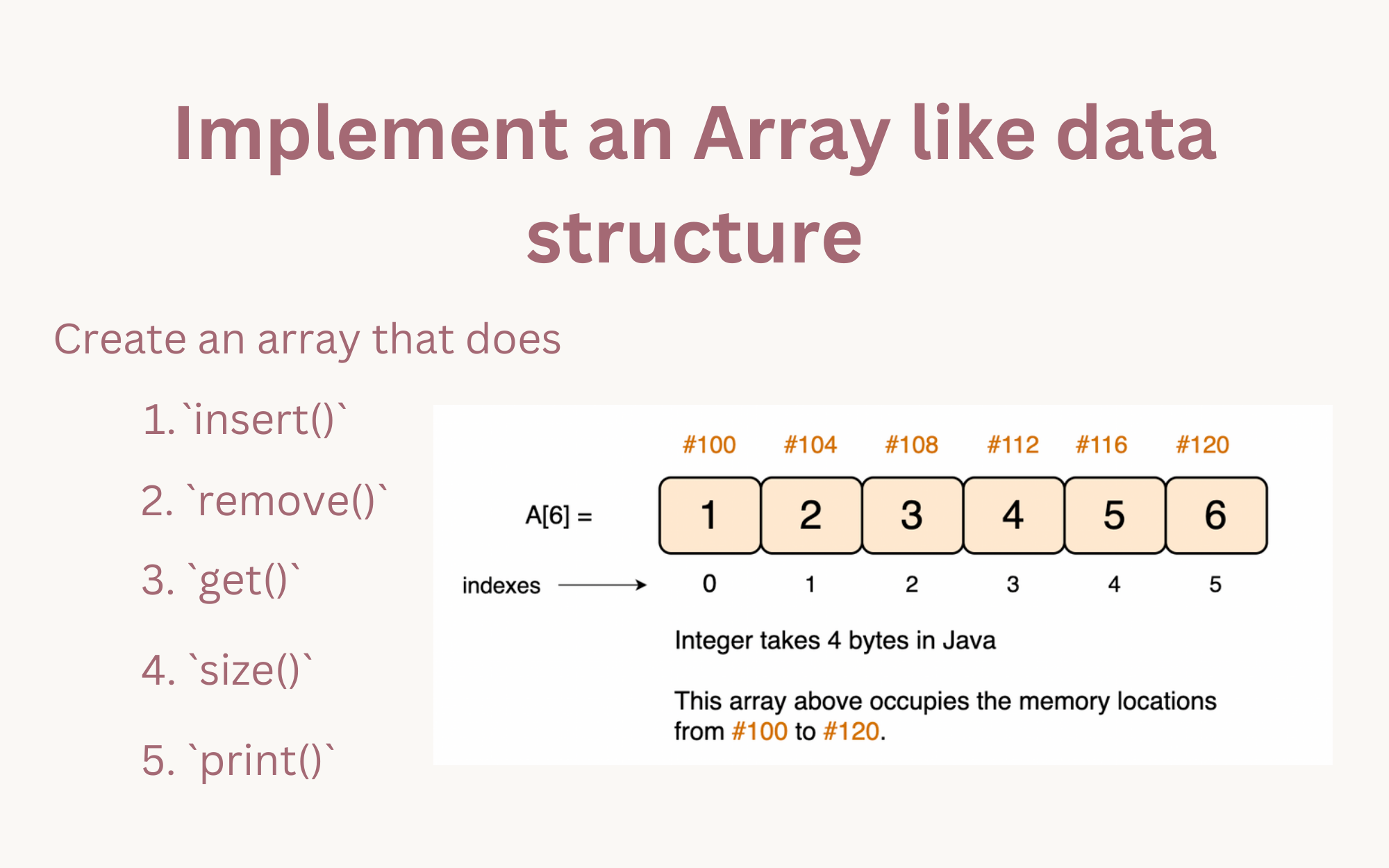
Two Sum Problem (Solutions in Java, JS, and TS)
In this two-sum problem, we use various approaches to solve the problem from brute force to an optimized approach. We also discuss the take-offs and talk about complexity analysis.
Solution Review: Get The First Set Bit Position Using the Left Shift
In the kth bit set/unset problem, we first write the algorithm, then some pseudocode, and then implement the solution.
Challenge 1: Get the First Set Bit Position Using the Left Shift
This problem is similar to the last lesson we discussed. If you need a clue, return to the previous lesson to further your understanding.
Check If Kth Bit Is Set/Unset Using Left Shift
In the kth bit set/unset problem, we need to write a program that checks whether the kth bit of a number is either 1 or 0.
Find the Bit Length of a Number
In this lesson, we use the Left Shift operator to find the bit length of a number. Introduction In this question, we take input and find its bit length. Problem Statement Given an input, find its bit length. Input: 8 Output: 4 (1000) Input: 2 Output: 2 (10) Input: 7
Solution Review: Missing Number
We solved the problem using lookup (hashtable), using the mathematical formula. Let's solve this more efficiently using bit-level operations with XOR and then optimize the solution.
Solution Review: Single Number
Single Number coding question, can be easily solved with XOR bitwise technique with linear time complexity.
Challenge 1: Single Number
In this lesson, every element appears twice except one element. We solve this using naive, and then we move to solve it more optimally using XOR operator.